c写一个控制器依据显示屏密码的启机限制功能,要求:控制器接收显示屏输入正确密码后解锁启机功能。
时间: 2024-02-11 12:07:10 浏览: 48
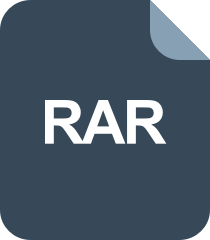
用C语言编写的开机密码

好的,我可以为您提供一个简单的示例代码,以便您更好地理解如何实现这个功能。
在本示例中,我们假设控制器和显示屏使用串口通信,并且密码为固定的字符串。您需要根据实际情况进行修改。
控制器代码:
```c
#include <stdio.h>
#include <string.h>
#include <stdbool.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define PASSWORD "123456" // 设定密码为123456
int main()
{
int fd;
struct termios options;
char buf[256];
bool unlocked = false; // 默认未解锁启机功能
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (fd < 0)
{
perror("open");
return -1;
}
// 配置串口
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~PARENB;
tcsetattr(fd, TCSANOW, &options);
// 初始化显示屏
write(fd, "init", 4);
while (true)
{
// 读取显示屏发送的数据
int n = read(fd, buf, 256);
if (n > 0)
{
buf[n] = '\0';
printf("Received: %s\n", buf);
// 判断是否输入了密码
if (strncmp(buf, PASSWORD, strlen(PASSWORD)) == 0)
{
// 输入的密码正确,解锁启机功能
unlocked = true;
printf("Password correct. Unlocked.\n");
write(fd, "unlock", 6);
}
else
{
printf("Password incorrect. Please try again.\n");
}
}
// 如果已经解锁启机功能,则退出循环,启动系统
if (unlocked)
{
break;
}
// 发送心跳信号
write(fd, "heartbeat", 9);
// 等待一段时间再继续执行,避免过于频繁地发送心跳信号
usleep(500000);
}
printf("System started.\n");
close(fd);
return 0;
}
```
显示屏代码:
```c
#include <stdio.h>
#include <string.h>
#include <stdbool.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define PASSWORD "123456" // 设定密码为123456
int main()
{
int fd;
struct termios options;
char buf[256];
bool password_entered = false;
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (fd < 0)
{
perror("open");
return -1;
}
// 配置串口
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~PARENB;
tcsetattr(fd, TCSANOW, &options);
// 初始化显示屏
write(fd, "init", 4);
while (true)
{
// 判断是否已经输入了密码
if (password_entered)
{
// 发送心跳信号
write(fd, "heartbeat", 9);
}
else
{
// 读取用户输入的密码
printf("Please enter password: ");
scanf("%s", buf);
if (strncmp(buf, PASSWORD, strlen(PASSWORD)) == 0)
{
// 输入的密码正确,发送给控制器进行验证
printf("Password correct. Sending to controller.\n");
write(fd, PASSWORD, strlen(PASSWORD));
password_entered = true;
}
else
{
printf("Password incorrect. Please try again.\n");
}
}
// 等待一段时间再继续执行,避免过于频繁地发送心跳信号
usleep(500000);
}
close(fd);
return 0;
}
```
在这个示例代码中,控制器和显示屏之间使用了串口通信,并且密码为固定的字符串。程序运行时,显示屏会不断等待用户输入密码,如果输入的密码正确,则发送给控制器进行验证,并在控制器解锁启机功能后退出循环,启动系统。如果输入的密码不正确,则显示屏会提示用户重新输入。控制器在接收到密码后进行验证,如果密码正确,则解锁启机功能,并向显示屏发送解锁指令,显示屏收到解锁指令后会显示相应的状态。
需要注意的是,本示例代码仅供参考,实际应用中需要根据具体的硬件平台和通信方式进行相应的修改和调试。
阅读全文
相关推荐
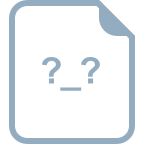
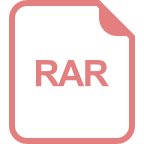
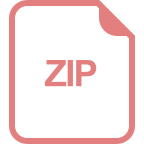
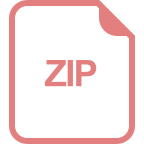
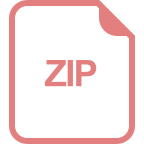
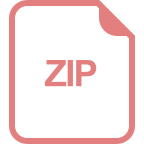
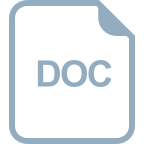
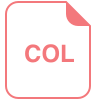
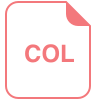
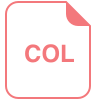
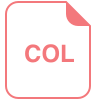
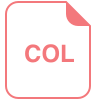
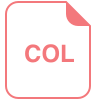
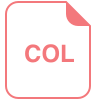
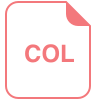
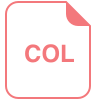
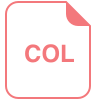
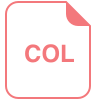