用vue写一个表单将查询数据通过mqtt下发并渲染到轮播表里
时间: 2024-05-03 09:23:31 浏览: 11
由于缺少具体的数据和mqtt服务信息,以下代码仅为示例代码,需要根据实际情况进行修改。
1. 安装所需依赖
```bash
npm install vue mqtt --save
```
2. 创建组件
```vue
<template>
<div>
<form @submit.prevent="handleSubmit">
<input type="text" v-model="query" placeholder="输入查询条件">
<button type="submit">查询</button>
</form>
<div v-if="loading">加载中...</div>
<div v-else-if="error">{{ error }}</div>
<div v-else>
<div v-for="(item, index) in data" :key="index">
<h3>{{ item.title }}</h3>
<p>{{ item.content }}</p>
</div>
<div v-if="data.length === 0">暂无数据</div>
<carousel :data="data"></carousel>
</div>
</div>
</template>
<script>
import mqtt from 'mqtt'
import Carousel from './Carousel.vue'
export default {
components: {
Carousel
},
data() {
return {
query: '',
loading: false,
error: '',
data: []
}
},
methods: {
handleSubmit() {
this.loading = true
this.error = ''
this.data = []
const client = mqtt.connect('wss://mqtt.example.com') // mqtt服务地址
client.on('connect', () => {
client.subscribe('query/result', (err) => {
if (err) {
this.loading = false
this.error = err.message
return
}
client.publish('query', JSON.stringify({ query: this.query }))
})
})
client.on('message', (topic, message) => {
if (topic === 'query/result') {
this.loading = false
this.data = JSON.parse(message.toString())
}
client.end()
})
}
}
}
</script>
```
3. 创建轮播表组件
```vue
<template>
<div>
<div v-if="data.length === 0">暂无数据</div>
<div v-else>
<div v-for="(item, index) in data" :key="index" v-show="currentIndex === index">
<h3>{{ item.title }}</h3>
<p>{{ item.content }}</p>
</div>
<div class="indicators">
<span v-for="(item, index) in data" :key="index" :class="{ active: currentIndex === index }" @click="handleChange(index)"></span>
</div>
</div>
</div>
</template>
<script>
export default {
props: {
data: {
type: Array,
default() {
return []
}
}
},
data() {
return {
currentIndex: 0
}
},
mounted() {
this.timer = setInterval(() => {
this.currentIndex++
if (this.currentIndex >= this.data.length) {
this.currentIndex = 0
}
}, 3000)
},
beforeDestroy() {
clearInterval(this.timer)
},
methods: {
handleChange(index) {
this.currentIndex = index
}
}
}
</script>
```
4. 在入口文件中注册组件
```js
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false
new Vue({
render: h => h(App)
}).$mount('#app')
```
5. 编写样式
```css
.indicators {
position: absolute;
bottom: 10px;
left: 50%;
transform: translateX(-50%);
display: flex;
}
.indicators span {
width: 10px;
height: 10px;
border-radius: 50%;
background-color: #999;
margin-right: 10px;
cursor: pointer;
transition: all 0.3s ease;
}
.indicators span.active {
background-color: #333;
transform: scale(1.2);
}
```
以上示例代码仅供参考,实际使用中还需要根据具体需求进行修改和完善。
相关推荐
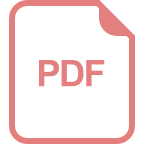
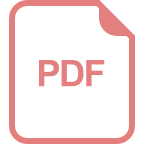
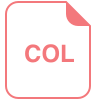
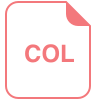
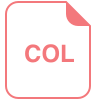
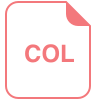
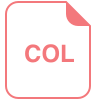









