语义分割.json转标签
时间: 2023-08-12 17:13:27 浏览: 99
将语义分割的结果保存在 JSON 文件中,需要将其转换成标签图像。以下是一种可能的方法:
1. 读取 JSON 文件,解析其中的数据。
2. 预处理数据,将像素坐标转换成标签图像中的索引。
3. 创建标签图像,将每个像素的标签设置为对应的类别。
4. 将标签图像保存为图像文件(如 PNG)。
下面是一个 Python 示例代码,可以实现将语义分割的 JSON 文件转换为标签图像:
```python
import json
import numpy as np
from PIL import Image
# 读取 JSON 文件
with open('segmentation.json', 'r') as f:
data = json.load(f)
# 解析数据
height, width = data['size']['height'], data['size']['width']
labels = np.zeros((height, width), dtype=np.uint8)
for obj in data['objects']:
label = obj['classTitle']
indices = np.array(obj['points']['exterior'], dtype=np.int32)
indices[:, 0] = np.clip(indices[:, 0], 0, width - 1)
indices[:, 1] = np.clip(indices[:, 1], 0, height - 1)
rr, cc = np.meshgrid(np.arange(height), np.arange(width), indexing='ij')
mask = np.logical_and.reduce(
(cc >= indices[:, 0].min(), cc <= indices[:, 0].max(),
rr >= indices[:, 1].min(), rr <= indices[:, 1].max()))
labels[mask] = label
# 创建标签图像
palette = {
'background': 0,
'object1': 1,
'object2': 2,
# 添加更多类别对应的颜色值
}
palette = np.array([palette[key] for key in palette], dtype=np.uint8)
image = Image.fromarray(palette[labels], mode='P')
image.putpalette(np.array([
0, 0, 0, # 背景
255, 0, 0, # 物体1
0, 255, 0, # 物体2
# 添加更多类别对应的颜色值
], dtype=np.uint8))
# 保存标签图像为 PNG 文件
image.save('segmentation.png')
```
上述代码假设 JSON 文件中每个对象的类别名称已经与标签值对应,可以根据需要进行修改。同时,上述代码也假设标签值为整数,如果需要浮点数类型的标签,可以调整数据类型和颜色映射表。
相关推荐
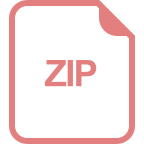
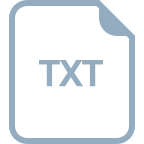
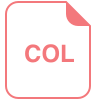













