请使用vue和bootstrap写一个能够自动插入和删除数据的表格(Table)
时间: 2024-05-02 12:17:10 浏览: 105
好的,以下是一个简单的使用 Vue 和 Bootstrap 实现自动插入和删除数据的表格:
```
<template>
<div>
<div class="form-inline mb-3">
<input type="text" class="form-control mr-2" placeholder="Name" v-model="newItem.name">
<input type="text" class="form-control mr-2" placeholder="Age" v-model="newItem.age">
<button class="btn btn-primary" @click="addNewItem">Add Item</button>
</div>
<table class="table">
<thead>
<tr>
<th>Name</th>
<th>Age</th>
<th></th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in items" :key="index">
<td>{{ item.name }}</td>
<td>{{ item.age }}</td>
<td>
<button class="btn btn-danger btn-sm" @click="removeItem(index)">Remove</button>
</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
export default {
data() {
return {
items: [
{ name: 'John', age: 25 },
{ name: 'Mary', age: 32 },
{ name: 'Bob', age: 45 }
],
newItem: { name: '', age: '' }
}
},
methods: {
addNewItem() {
this.items.push(this.newItem)
this.newItem = { name: '', age: '' }
},
removeItem(index) {
this.items.splice(index, 1)
}
}
}
</script>
```
在上面的代码中,我们定义了一个 `items` 数组来存储表格中的数据,以及一个 `newItem` 对象来存储用户输入的新数据。在模板中,我们使用 Bootstrap 的表单和表格组件来渲染界面,并使用 `v-for` 指令遍历 `items` 数组来动态生成表格行。在用户输入新数据后,我们使用 `addNewItem` 方法将其添加到 `items` 数组中,并清空 `newItem` 对象。在删除数据时,我们使用 `removeItem` 方法根据索引从 `items` 数组中删除对应的数据。
这个示例只是一个简单的演示,你可以根据需要对其进行扩展和优化。
阅读全文
相关推荐
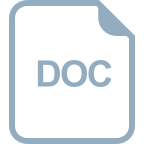
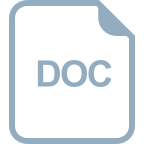
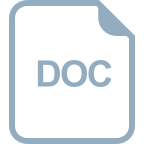
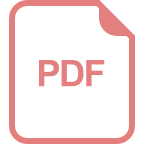
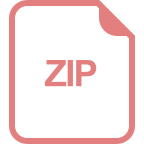
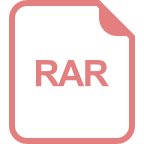
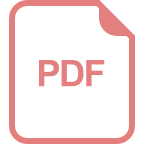
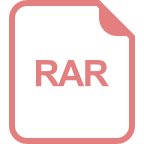
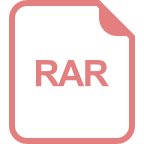
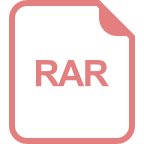
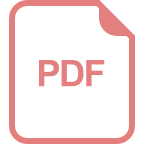
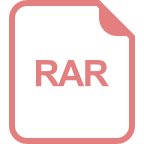
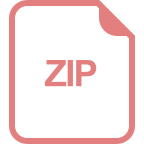
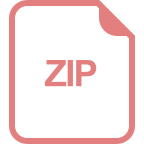
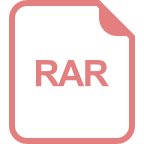
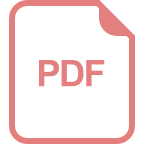
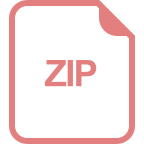
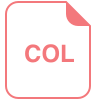
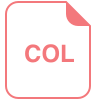