可视化实现粒子群优化算法的代码
时间: 2023-10-26 20:20:42 浏览: 99
由于粒子群优化算法的实现方式较为复杂,且需要涉及到较多的数学知识,因此在此不能提供完整的代码实现。但是,可以提供一个简单的可视化实现粒子群优化算法的示例,供参考:
```python
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
# 定义目标函数
def func(x):
return np.sin(x) + np.sin(10 * x / 3)
# 粒子群优化算法
def PSO(func, x_min, x_max, n_particles=50, max_iter=100, w=0.8, c1=2.0, c2=2.0):
# 初始化粒子群
x = np.random.uniform(x_min, x_max, n_particles)
v = np.zeros_like(x)
p = x.copy()
p_best = p.copy()
f_best = np.min(func(p_best))
# 迭代
for i in range(max_iter):
# 更新速度和位置
v = w * v + c1 * np.random.rand() * (p_best - p) + c2 * np.random.rand() * (np.min(p_best) - p)
x = x + v
# 防止粒子越界
x[x < x_min] = x_min
x[x > x_max] = x_max
# 更新个体最优位置和全局最优位置
f = func(x)
mask = f < func(p_best)
p_best[mask] = x[mask]
f_best = np.min(func(p_best))
yield x, p_best, f_best
# 可视化
fig, ax = plt.subplots()
ax.set_xlim(-10, 10)
ax.set_ylim(-3, 3)
line, = ax.plot([], [], 'bo')
p_best_line, = ax.plot([], [], 'ro')
f_best_text = ax.text(-9, 2.5, '', fontsize=12)
def init():
line.set_data([], [])
p_best_line.set_data([], [])
f_best_text.set_text('')
return line, p_best_line, f_best_text
def update(data):
x, p_best, f_best = data
line.set_data(x, func(x))
p_best_line.set_data(p_best, func(p_best))
f_best_text.set_text('Global Best: {:.6f}'.format(f_best))
return line, p_best_line, f_best_text
ani = FuncAnimation(fig, update, frames=PSO(func, -10, 10), init_func=init, blit=True)
plt.show()
```
在上面的代码中,定义了一个目标函数 `func`,并通过粒子群优化算法来最小化该函数。具体实现过程如下:
1. 定义粒子群的个数、最大迭代次数、惯性权重、加速常数等参数,以及粒子的初始位置、速度和个体最优位置。
2. 每次迭代,根据当前速度和位置更新粒子的位置,同时更新个体最优位置和全局最优位置。
3. 将每一次迭代得到的粒子位置、个体最优位置和全局最优位置进行可视化。
4. 最终输出全局最优位置和最优解。
需要注意的是,上述代码中的粒子群优化算法实现方式较为简单,仅供参考,实际应用中可能需要根据具体问题进行调整。
阅读全文
相关推荐
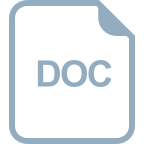
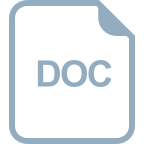
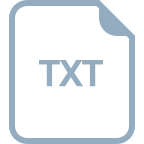
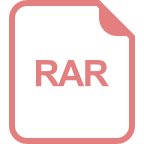
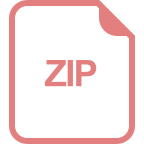
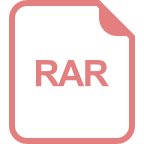
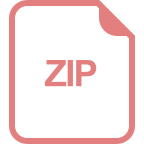
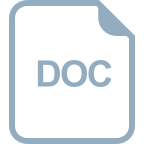
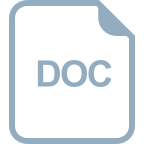
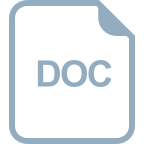
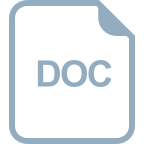
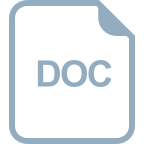
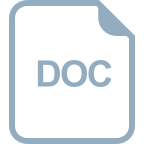
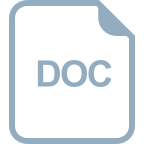
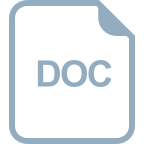
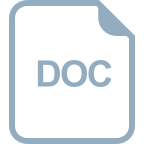