Fl_Multiline_Input产生的字符串数组如何使用atof进行转换和遍历的实例
时间: 2023-05-28 20:05:31 浏览: 77
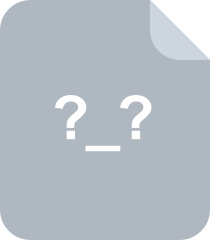
通过for实现了数组和字符串的遍历
假设我们有一个Fl_Multiline_Input控件,并且用户在其中输入了一些数字,每个数字之间用换行符分隔。我们想使用atof函数将这些数字转换为浮点数,并将它们存储在一个数组中。
首先,我们需要获取Fl_Multiline_Input控件中的文本,并将其分割成单独的行。我们可以使用Fl_Multiline_Input的value()方法来获取文本,然后使用字符串流(stringstream)来将文本分割成行:
```cpp
#include <FL/Fl.H>
#include <FL/Fl_Window.H>
#include <FL/Fl_Multiline_Input.H>
#include <sstream>
#include <vector>
int main() {
Fl_Window window(300, 200, "Example");
Fl_Multiline_Input input(10, 10, 280, 180);
window.end();
window.show();
while (window.shown()) {
Fl::wait();
if (input.changed()) {
std::string text = input.value();
std::stringstream ss(text);
std::vector<std::string> lines;
std::string line;
while (std::getline(ss, line)) {
lines.push_back(line);
}
// Convert lines to floats
std::vector<float> floats;
for (auto& line : lines) {
float f = std::atof(line.c_str());
floats.push_back(f);
}
// Print floats
for (auto& f : floats) {
std::cout << f << std::endl;
}
}
}
return 0;
}
```
在上面的代码中,我们使用了一个while循环来等待窗口关闭。在每次循环中,我们检查Fl_Multiline_Input控件是否发生了变化(即用户是否输入了新的文本)。如果输入发生了变化,我们获取输入的文本,将其分割成行,并将每行文本转换为浮点数。
我们使用了一个vector来存储每行文本和每个浮点数。这使得我们可以轻松地遍历它们并执行任何操作。在上面的代码中,我们只是简单地将每个浮点数打印到控制台。
注意,我们使用了std::atof函数来将字符串转换为浮点数。这个函数接受一个const char*参数,因此我们需要使用c_str()方法来将std::string转换为const char*。
此代码示例仅用于演示目的。在实际应用中,您需要添加错误检查和异常处理以确保输入的文本是有效的浮点数。
阅读全文
相关推荐
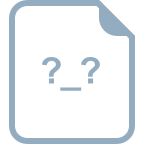
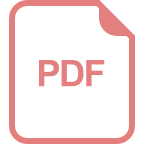






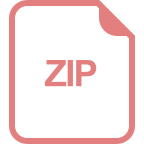
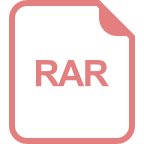
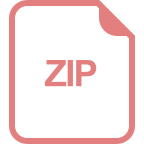
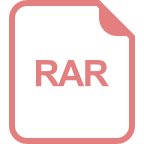
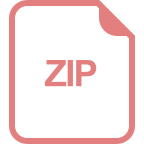
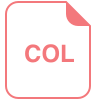



