用springboot帮我写一个文件上传的功能
时间: 2024-05-07 11:16:59 浏览: 10
好的,基于Spring Boot,我们可以使用Spring Boot Starter Web 和 Spring Boot Starter Thymeleaf依赖来实现文件上传功能。以下是一个示例代码:
首先,在Maven或Gradle中添加以下依赖:
```xml
<!-- Spring Boot Starter Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Boot Starter Thymeleaf -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
```
接着,在application.properties文件中添加以下配置:
```properties
# 设置文件上传限制
spring.servlet.multipart.max-file-size=10MB
spring.servlet.multipart.max-request-size=10MB
```
最后,编写一个Controller,处理文件上传请求:
```java
@Controller
public class FileUploadController {
@GetMapping("/")
public String index() {
return "upload";
}
@PostMapping("/upload")
public String singleFileUpload(@RequestParam("file") MultipartFile file, RedirectAttributes redirectAttributes) {
if (file.isEmpty()) {
redirectAttributes.addFlashAttribute("message", "Please select a file to upload");
return "redirect:/";
}
try {
// 保存文件到指定路径
byte[] bytes = file.getBytes();
Path path = Paths.get("/path/to/upload/folder/" + file.getOriginalFilename());
Files.write(path, bytes);
redirectAttributes.addFlashAttribute("message", "File uploaded successfully");
} catch (IOException e) {
e.printStackTrace();
}
return "redirect:/";
}
}
```
在上面的代码中,我们使用了两个路由:/和/upload。/用于返回上传页面(upload.html),upload用于接收文件上传请求并保存文件。
在upload.html中,我们可以添加如下HTML代码:
```html
<!doctype html>
<html>
<head>
<title>File Upload</title>
</head>
<body>
<h1>File Upload</h1>
<form method="POST" action="/upload" enctype="multipart/form-data">
<div>
<input type="file" name="file"/>
</div>
<br/>
<div>
<button type="submit">Upload</button>
</div>
</form>
<div th:if="${message}">
<p th:text="${message}"></p>
</div>
</body>
</html>
```
这样,我们就完成了基于Spring Boot的文件上传功能实现。当然,你也可以使用其他框架或工具来实现文件上传功能。
相关推荐
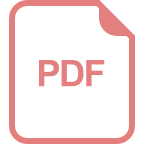














