采用Vc+Qt混合编程,实现字节填充算法
时间: 2023-05-28 11:03:03 浏览: 147
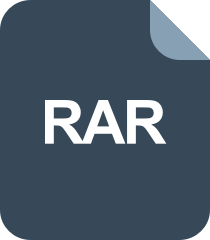
cv.rar_VC与matlab 混合 编程实现
下面是一个基于Vc Qt混合编程实现字节填充算法的示例代码:
```
#include <QtWidgets/QApplication>
#include <Vc/Vc>
using namespace Vc;
// 定义字节填充算法
template<typename T>
void byteFill(T *dst, const T *src, const T *pattern, size_t count)
{
if (count == 0) {
return;
}
const T *end = dst + count;
const auto *vsrc = reinterpret_cast<const simd<T> *>(src);
const auto *vpattern = reinterpret_cast<const simd<T> *>(pattern);
auto *vdst = reinterpret_cast<simd<T> *>(dst);
// 按照Vc的SIMD宽度(默认为16)进行循环
do {
*vdst++ = (*vsrc++) | *vpattern;
} while (vdst < reinterpret_cast<simd<T> *>(end));
// 处理剩余的不足一个SIMD宽度的部分
if (reinterpret_cast<const T *>(vdst) < end) {
const T *tailSrc = reinterpret_cast<const T *>(vsrc);
const T *tailPattern = reinterpret_cast<const T *>(vpattern);
T *tailDst = reinterpret_cast<T *>(vdst);
do {
*tailDst++ = (*tailSrc++) | *tailPattern++;
} while (tailDst < end);
}
}
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
// 测试代码
int src[] = {0xdeadbeef, 0x12345678, 0xabcdef12};
int pattern[] = {0x55555555, 0xaaaaaaaa, 0xcccccccc};
int dst[sizeof(src) / sizeof(int)];
byteFill(dst, src, pattern, sizeof(src) / sizeof(int));
for (int i = 0; i < sizeof(src) / sizeof(int); i++) {
qDebug("%08x", dst[i]);
}
return a.exec();
}
```
该代码使用了Vc库中的`simd`模板类和`reinterpret_cast`进行指针类型转换,实现了对指定内存区域的字节填充操作。在主函数中,我们对该函数进行了简单的测试,输出了填充后的结果。
阅读全文
相关推荐
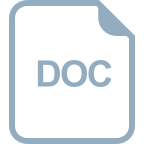
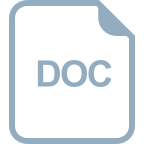
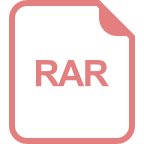
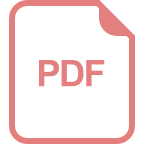
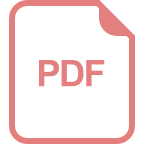
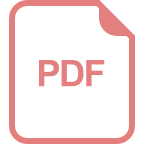
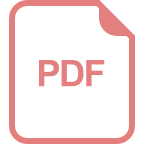
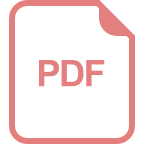
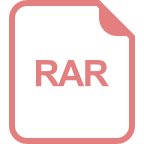
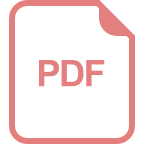
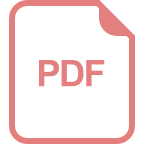
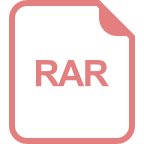
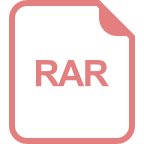
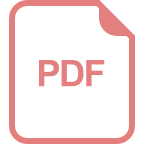
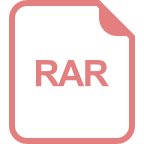
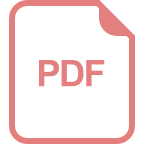