<nut-col :span="12" style="background-color: #1de9b6"> <router-link to="/rewards/rewardslist"> <i class="fa fa-gift fa-fw" aria-hidden="true"></i> <span class="info"> <strong>{{ rewards }}</strong> <span>我的嘉奖</span> </span> </router-link> </nut-col> </nut-row>发送ajax获取rewards值
时间: 2023-11-16 17:17:00 浏览: 183
可以使用 Vue 的生命周期钩子函数 `created` 来发送 AJAX 请求,并将获取到的 `rewards` 值保存在 Vue 实例的数据模型中。代码示例如下:
```javascript
new Vue({
el: '#app',
data: {
rewards: 0,
},
created() {
// 发送 AJAX 请求
axios.get('/api/rewards')
.then(response => {
this.rewards = response.data;
})
.catch(error => {
console.error(error);
});
},
});
```
在上面的代码中,我们使用 Axios 库发送 GET 请求,请求地址为 `/api/rewards`。当请求成功时,我们将服务器返回的数据保存在 `rewards` 变量中,这样就可以在模板中使用 `{{ rewards }}` 来显示当前用户的嘉奖数量了。
需要注意的是,上面的代码中使用了箭头函数来避免 `this` 指向出错的问题。如果你使用的是普通函数,则需要在 `created` 函数中使用 `var self = this;` 来保存 `this` 的值,然后在回调函数中使用 `self` 来访问 Vue 实例的数据模型。
相关问题
<nut-cell title="工作写实" :is-link="true" to="/realistic/list" :show-icon="true" />怎么传值
你可以通过 props 属性来传值。在父组件中使用子组件时,通过设置子组件的 props 属性,将数据传递给子组件。在子组件中,可以通过 this.$props 访问这些属性。以下是一个示例:
```html
<template>
<nut-cell :title="title" :is-link="isLink" :to="to" :show-icon="showIcon" />
</template>
<script>
import NutCell from "@/components/NutCell.vue";
export default {
name: "ParentComponent",
components: {
NutCell,
},
data() {
return {
title: "工作写实",
isLink: true,
to: "/realistic/list",
showIcon: true,
};
},
};
</script>
```
在上面的示例中,我们通过 props 属性将 title、isLink、to 和 showIcon 传递给 NutCell 组件。在 NutCell 组件中,我们可以通过 this.$props 访问这些属性。
<nut-cell title="工作写实" :is-link="true" to="/realistic/list" :show-icon="true" />怎么传值,怎么取值
你可以使用props来传递值,例如:
```html
<!-- 父组件 -->
<template>
<div>
<nut-cell :title="title" :is-link="isLink" :show-icon="showIcon" />
</div>
</template>
<script>
import NutCell from '@/components/NutCell.vue'
export default {
components: {
NutCell
},
data() {
return {
title: '工作写实',
isLink: true,
showIcon: true
}
}
}
</script>
```
你可以在子组件中使用props来接收这些值,例如:
```html
<!-- 子组件 NutCell.vue -->
<template>
<div class="nut-cell">
<div class="nut-cell__title">{{ title }}</div>
<div class="nut-cell__right-icon" v-if="showIcon">
<i class="iconfont icon-right"></i>
</div>
</div>
</template>
<script>
export default {
props: {
title: {
type: String,
default: ''
},
isLink: {
type: Boolean,
default: false
},
showIcon: {
type: Boolean,
default: false
}
}
}
</script>
```
这样就能够在父组件中传递数据给子组件了,子组件可以根据props来渲染自己的内容。对于父组件来说,可以使用computed或者methods来获取子组件的数据,例如:
```html
<!-- 父组件 -->
<template>
<div>
<nut-cell ref="cell" :title="title" :is-link="isLink" :show-icon="showIcon" />
<button @click="handleClick">获取子组件数据</button>
</div>
</template>
<script>
import NutCell from '@/components/NutCell.vue'
export default {
components: {
NutCell
},
data() {
return {
title: '工作写实',
isLink: true,
showIcon: true
}
},
methods: {
handleClick() {
const title = this.$refs.cell.title
const isLink = this.$refs.cell.isLink
const showIcon = this.$refs.cell.showIcon
console.log(title, isLink, showIcon)
}
}
}
</script>
```
在这个例子中,我们通过在父组件中给子组件添加ref属性来获取子组件的实例,并且通过访问子组件的属性来获取子组件的数据。
阅读全文
相关推荐
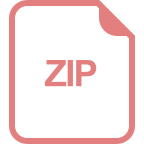
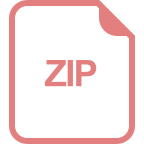
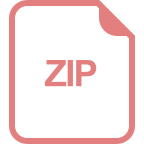
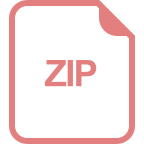
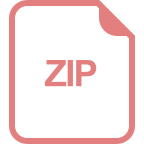
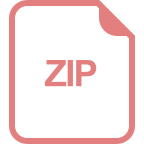
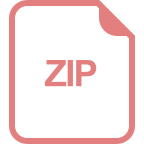
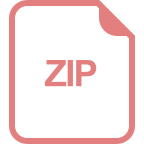
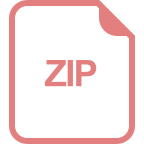
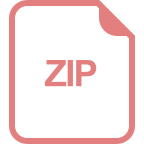
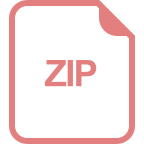
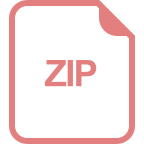
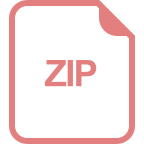
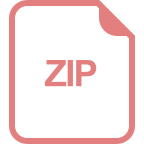
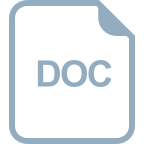
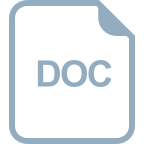
