写一段A星算法
时间: 2023-07-10 10:18:31 浏览: 37
下面是一个简单的伪代码实现A星算法的例子:
```
function AStar(start, goal) {
// 初始化起始节点的f值、g值和h值
start.f = 0;
start.g = 0;
start.h = heuristic(start, goal);
// 初始化开放列表和关闭列表
openList = [start];
closedList = [];
while (openList.length > 0) {
// 从开放列表中选择f值最小的节点
currentNode = openList[0];
for (i = 1; i < openList.length; i++) {
if (openList[i].f < currentNode.f) {
currentNode = openList[i];
}
}
// 如果当前节点是目标节点,则返回路径
if (currentNode == goal) {
return getPath(currentNode);
}
// 将当前节点从开放列表中移除,并加入关闭列表
index = openList.indexOf(currentNode);
openList.splice(index, 1);
closedList.push(currentNode);
// 扩展当前节点的所有邻居
neighbors = getNeighbors(currentNode);
for (i = 0; i < neighbors.length; i++) {
neighbor = neighbors[i];
// 如果邻居节点已经在关闭列表中,则跳过
if (closedList.includes(neighbor)) {
continue;
}
// 计算邻居节点的g值和h值
tentativeG = currentNode.g + distanceBetween(currentNode, neighbor);
tentativeH = heuristic(neighbor, goal);
// 如果邻居节点不在开放列表中,则加入开放列表
if (!openList.includes(neighbor)) {
neighbor.g = tentativeG;
neighbor.h = tentativeH;
neighbor.f = neighbor.g + neighbor.h;
neighbor.parent = currentNode;
openList.push(neighbor);
}
// 如果邻居节点在开放列表中,并且新的g值更小,则更新g值和父节点
else if (tentativeG < neighbor.g) {
neighbor.g = tentativeG;
neighbor.f = neighbor.g + neighbor.h;
neighbor.parent = currentNode;
}
}
}
// 如果开放列表为空,表示无法到达目标节点
return null;
}
```
需要注意的是,上述代码中的heuristic函数和distanceBetween函数需要根据具体情况进行实现,以保证算法的正确性和效率。同时,getPath函数可以通过回溯每个节点的父节点来构造最短路径。
相关推荐
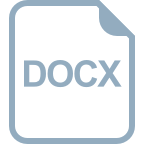
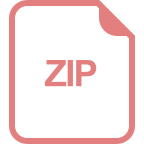
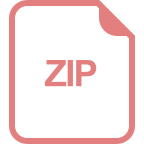














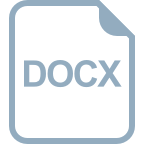