用tensorflow写一个图像内容识别的代码
时间: 2023-05-12 10:05:06 浏览: 109
好的,以下是一个使用 TensorFlow 实现图像内容识别的代码示例:
```python
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
# 加载数据集
mnist = tf.keras.datasets.mnist
(train_images, train_labels), (test_images, test_labels) = mnist.load_data()
# 数据预处理
train_images = train_images / 255.0
test_images = test_images / 255.0
# 定义模型
model = tf.keras.Sequential([
tf.keras.layers.Flatten(input_shape=(28, 28)),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(10)
])
# 编译模型
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
# 训练模型
model.fit(train_images, train_labels, epochs=10)
# 评估模型
test_loss, test_acc = model.evaluate(test_images, test_labels, verbose=2)
print('\nTest accuracy:', test_acc)
# 预测结果
probability_model = tf.keras.Sequential([model, tf.keras.layers.Softmax()])
predictions = probability_model.predict(test_images)
# 可视化预测结果
def plot_image(i, predictions_array, true_label, img):
predictions_array, true_label, img = predictions_array[i], true_label[i], img[i]
plt.grid(False)
plt.xticks([])
plt.yticks([])
plt.imshow(img, cmap=plt.cm.binary)
predicted_label = np.argmax(predictions_array)
if predicted_label == true_label:
color = 'blue'
else:
color = 'red'
plt.xlabel("{} {:2.0f}% ({})".format(predicted_label,
100 * np.max(predictions_array),
true_label),
color=color)
def plot_value_array(i, predictions_array, true_label):
predictions_array, true_label = predictions_array[i], true_label[i]
plt.grid(False)
plt.xticks(range(10))
plt.yticks([])
thisplot = plt.bar(range(10), predictions_array, color="#777777")
plt.ylim([0, 1])
predicted_label = np.argmax(predictions_array)
thisplot[predicted_label].set_color('red')
thisplot[true_label].set_color('blue')
# 随机选择一张测试图片进行预测
i = np.random.randint(0, len(test_images))
plt.figure(figsize=(6,3))
plt.subplot(1,2,1)
plot_image(i, predictions, test_labels, test_images)
plt.subplot(1,2,2)
plot_value_array(i, predictions, test_labels)
plt.show()
```
这个代码使用了 TensorFlow 框架来实现图像内容识别,使用的数据集是 MNIST 手写数字数据集。模型使用了两个全连接层,其中第一个层使用了 ReLU 激活函数,第二个层没有使用激活函数。模型的输出是一个长度为 10 的向量,表示每个数字的概率。模型使用了 Softmax 函数将输出转换为概率分布。在训练过程中,使用了 Adam 优化器和交叉熵损失函数。最终,我们使用测试集对模型进行了评估,并随机选择了一张测试图片进行了预测。
相关推荐
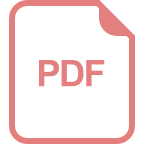
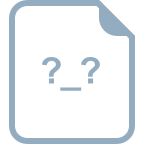
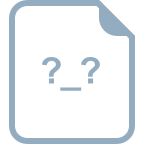














