用python从文件in.txt中读取内容,将其中的奇数行写入文件out.txt
时间: 2024-05-15 19:14:11 浏览: 228
可以使用Python中的内置函数open()来打开文件,然后使用循环和条件语句来读取和筛选内容,最后将符合条件的内容写入到另一个文件中。
以下是示例代码:
```python
with open('in.txt', 'r') as in_file, open('out.txt', 'w') as out_file:
# 使用with语句打开文件,in_file为输入文件,out_file为输出文件
# 'r'和'w'分别表示读和写的模式
lines = in_file.readlines() # 读取所有行
for i in range(len(lines)):
if i % 2 == 0: # 判断是否为奇数行
out_file.write(lines[i]) # 将符合条件的行写入输出文件
```
注意,这里假设in.txt中的每一行都以换行符`\n`结尾,如果文件行末没有换行符,可能会导致读取错误。
相关问题
从文件in.txt中读取内容,将其中的奇数行写入文件out.txt
好的,以下是Python代码实现:
```python
with open('in.txt', 'r') as f_in, open('out.txt', 'w') as f_out:
lines = f_in.readlines()
for i in range(1, len(lines), 2): # 从第1行开始,步长为2,即取奇数行
f_out.write(lines[i])
```
首先使用`open()`函数打开输入文件`in.txt`和输出文件`out.txt`,分别以只读模式和写入模式打开。
接着使用`readlines()`方法读取输入文件中的所有行,并存储在一个列表中。
然后使用`range()`函数和步长为2的方式,来获取奇数行的索引位置,即`1, 3, 5, ...`,并使用`write()`方法将这些行写入输出文件中。
最后,使用`with`语句可以确保文件的正确关闭。
从文件demo.xt中读取内容,将其中的奇数行写入文件 python
好的,这里是Python代码实现从文件demo.txt中读取内容,将其中的奇数行写入文件的方法:
```python
with open('demo.txt', 'r') as f:
lines = f.readlines()
with open('output.txt', 'w') as f:
for i in range(len(lines)):
if i % 2 == 0:
f.write(lines[i])
```
首先,我们使用`with open('demo.txt', 'r') as f`语句打开demo.txt文件,并使用`f.readlines()`方法将文件中的所有内容读取到一个列表中。然后,我们使用`with open('output.txt', 'w') as f`语句打开一个名为output.txt的文件,并以写入模式写入奇数行。
在`for`循环中,我们使用`range(len(lines))`生成一个0到`len(lines)-1`的数字序列,表示文件中每一行的索引。如果当前行的索引是偶数,我们就使用`f.write(lines[i])`语句将该行写入output.txt文件中。
希望能够帮助到你!
阅读全文
相关推荐













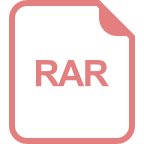