用python写一个定时启动某程序的代码
时间: 2023-08-22 07:05:50 浏览: 108
好的,下面是用Python写定时启动某程序的代码:
```python
import os
import time
# 设置等待时间
wait_time = 3600 # 单位为秒,这里设置为1个小时
# 设置要启动的程序路径
program_path = 'C:\\Program Files\\xxx\\program.exe'
while True:
# 获取当前时间
current_time = time.localtime()
# 判断是否为每天的9点启动程序时间,如果是则启动
if current_time.tm_hour == 9 and current_time.tm_min == 0 and current_time.tm_sec == 0:
os.startfile(program_path) # 启动程序
# 等待一段时间
time.sleep(wait_time)
```
这段代码中,我们首先设置了等待时间wait_time,也就是每次循环时等待的时间。然后,我们设置了要启动的程序路径program_path。
在无限循环中,我们首先获取当前时间。如果当前时间为每天的9点0分0秒,则启动程序。然后,我们等待一段时间,等待下次循环。
这样,我们的程序就可以定时启动某个程序了。
相关问题
使用python写一个微信定时推送的小程序
抱歉,作为一个AI语言模型,我不能在本地运行代码。不过,我可以提供一个基本思路和代码示例,供您参考。请注意,由于微信官方限制,目前无法使用第三方程序向微信推送消息。
基本思路:
1.使用itchat库登录微信账号
2.编写消息推送函数
3.使用time库实现定时功能
4.使用多线程或协程实现后台运行
代码示例:
```python
import itchat
import time
# 登录微信账号
itchat.auto_login()
# 消息推送函数
def send_msg():
itchat.send('Hello, World!', toUserName='filehelper') # 向文件传输助手发送消息
# 定时任务函数
def task():
while True:
now = time.localtime()
if now.tm_hour == 8 and now.tm_min == 0: # 每天早上8点推送消息
send_msg()
time.sleep(60) # 等待1分钟
# 后台运行
if __name__ == '__main__':
t = threading.Thread(target=task)
t.start()
```
说明:
1. 在上述示例中,我们使用了itchat库,该库可以模拟登录微信账号,并提供发送消息的功能。
2. send_msg函数中使用了itchat.send函数向文件传输助手发送了一条消息。
3. task函数中使用了time库的localtime函数获取当前时间,并判断是否到了定时推送的时间。如果是,则调用send_msg函数发送消息。
4. 最后使用多线程或协程的方式实现后台运行。在本例中,我们创建了一个线程,并将task函数作为其target参数传入,然后调用start函数启动该线程。
写个python脚本,定时启动mac上的程序
### 回答1:
你可以使用crontab来实现定时启动Mac上的程序。 你可以在终端中输入 crontab -e 命令,然后在文本编辑器中输入以下内容: * * * * * /path/to/program这将在每分钟都运行/path/to/program。你也可以根据自己的需要更改运行时间,例如每小时运行一次,每天运行一次等等。
### 回答2:
要编写一个Python脚本,用于定时启动Mac上的程序,可以使用Python的内置模块`os`和`time`来实现。下面是一个简单的示例代码:
```python
import os
import time
def start_program(program_path, delay):
# 等待指定的延迟时间
time.sleep(delay)
# 使用os模块执行命令,启动指定路径下的程序
os.system(f"open {program_path}")
if __name__ == "__main__":
# 设置程序路径和延迟时间
program_path = "/Applications/TextEdit.app" # 修改为要启动的程序的实际路径
delay = 10 # 延迟时间,单位为秒
# 调用函数启动程序
start_program(program_path, delay)
```
在上面的示例代码中,`start_program`函数接受两个参数:`program_path`指定要启动的程序路径,`delay`表示延迟时间,即脚本运行后等待一段时间再启动程序。这里使用`time.sleep`函数来实现延迟。
在`start_program`函数中,使用`os.system`函数执行命令`open`来启动指定路径下的程序。这个示例中使用的是TextEdit.app作为示例,你可以根据需要修改`program_path`为你想要启动的程序的实际路径。
最后,在`if __name__ == "__main__":`语句中,设置了程序路径和延迟时间,并调用`start_program`函数来启动程序。
你可以将以上代码保存为一个Python脚本文件,比如`start_program.py`,然后在终端中运行该脚本(例如`python start_program.py`),程序将在延迟时间后启动。
### 回答3:
要编写一个 Python 脚本以定时启动 Mac 上的程序,可以使用 `os` 模块来执行系统命令。以下是一个实例:
```python
import os
import time
def start_program():
program_path = "/Applications/Calculator.app" # 替换为要启动的程序的路径或命令
os.system(f"open {program_path}")
def main():
interval = 3600 # 以秒为单位设置时间间隔,这里设置为一小时
while True:
start_program()
time.sleep(interval)
if __name__ == "__main__":
main()
```
在上面的例子中,`start_program` 函数用来启动指定的程序。你需要将 `program_path` 变量替换为实际程序的路径或命令。例如,这里我用的是计算器应用程序。
`main` 函数会在一个死循环中定时调用 `start_program` 函数。时间间隔可以通过 `interval` 变量来调整,这里设置为一个小时(3600秒)。
这个脚本会不断地启动程序,然后等待指定的时间间隔后再次启动。可以使用 `Ctrl + C` 组合键来中断脚本的运行。
请注意,为了能够在 Mac 上执行该脚本,你需要安装 Python,然后通过终端运行该脚本。
阅读全文
相关推荐
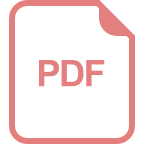
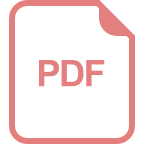
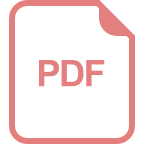













