编写一个函数,由实参传来一个字符串,统计此字符串中字母、数字、空格和其他字符的个数
时间: 2023-11-17 15:11:17 浏览: 85
以下是Python代码实现:
```python
def count_chars(s):
num_letters = 0
num_digits = 0
num_spaces = 0
num_others = 0
for c in s:
if c.isalpha():
num_letters += 1
elif c.isdigit():
num_digits += 1
elif c.isspace():
num_spaces += 1
else:
num_others += 1
return num_letters, num_digits, num_spaces, num_others
```
这个函数接受一个字符串参数`s`,并创建变量`num_letters`、`num_digits`、`num_spaces`和`num_others`,初始值都为0。然后,它遍历字符串`s`中的每个字符,检查它是字母、数字、空格还是其他字符,并相应地增加适当的计数器。最后,函数返回一个元组,其中包含了四个计数器的值。
相关问题
编写一个函数由实参传来一个字符串统计此字符串中字母数字空格和其他字符的个数在主函数中输入字符串以及输出上述的结果
以下是 Python 代码实现:
```python
def count_chars(string):
letters = 0
digits = 0
spaces = 0
others = 0
for char in string:
if char.isalpha():
letters += 1
elif char.isdigit():
digits += 1
elif char.isspace():
spaces += 1
else:
others += 1
print("字母个数:", letters)
print("数字个数:", digits)
print("空格个数:", spaces)
print("其他字符个数:", others)
# 主函数
if __name__ == '__main__':
string = input("请输入一个字符串: ")
count_chars(string)
```
示例输出:
```
请输入一个字符串: Hello World! 123
字母个数: 10
数字个数: 3
空格个数: 2
其他字符个数: 1
```
由C语言实现编写一个函数由实参传来一个字符串统计此字符串中字母数字空格和其他字符的个数在主函数中输入字符串以及输出上述的结果
下面是一个简单的C语言函数,可以统计一个字符串中字母、数字、空格和其他字符的个数:
```c
#include <stdio.h>
#include <ctype.h>
void count_chars(char *str, int *letters, int *digits, int *spaces, int *others) {
char ch;
*letters = *digits = *spaces = *others = 0;
while ((ch = *str++) != '\0') {
if (isalpha(ch))
(*letters)++;
else if (isdigit(ch))
(*digits)++;
else if (isspace(ch))
(*spaces)++;
else
(*others)++;
}
}
int main() {
char str[100];
int letters, digits, spaces, others;
printf("Enter a string: ");
fgets(str, sizeof(str), stdin);
count_chars(str, &letters, &digits, &spaces, &others);
printf("Letters: %d\n", letters);
printf("Digits: %d\n", digits);
printf("Spaces: %d\n", spaces);
printf("Other characters: %d\n", others);
return 0;
}
```
在主函数中,我们首先输入一个字符串,然后调用 `count_chars` 函数来统计字符的个数。函数使用 `ctype.h` 中的 `isalpha`、`isdigit` 和 `isspace` 函数来判断字符的类型。在函数中,我们使用指针来返回每种类型字符的个数,因为需要修改这些变量的值。在主函数中,我们只需要打印这些变量的值即可。
阅读全文
相关推荐












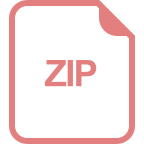