根据文件路径filepath 文件内容String类型的content 文件名称fileName 使用java转成doc文档 要是多张要带页码
时间: 2024-02-05 12:13:33 浏览: 68
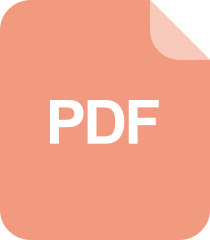
Android中Java根据文件头获取文件类型的方法
要将文件内容转化成doc文档,需要使用Apache POI库来实现。以下是一个Java代码示例:
```java
import java.io.File;
import java.io.FileOutputStream;
import org.apache.poi.hwpf.HWPFDocument;
import org.apache.poi.hwpf.usermodel.Range;
public class ConvertToDoc {
public static void main(String[] args) throws Exception {
String filepath = "example.txt";
String content = "This is an example text.";
String fileName = "example.doc";
// Create a new Word document
HWPFDocument document = new HWPFDocument();
Range range = document.getRange();
// Insert the content into the document
range.insertAfter(content);
// Save the document to a file
FileOutputStream out = new FileOutputStream(new File(fileName));
document.write(out);
out.close();
}
}
```
如果要将多个文件转换成多页的doc文档,可以先将每个文件转换成单页的doc文档,然后再将这些单页文档合并成一个多页文档。以下是一个Java代码示例:
```java
import java.io.File;
import java.io.FileOutputStream;
import java.util.ArrayList;
import java.util.List;
import org.apache.poi.hwpf.HWPFDocument;
import org.apache.poi.hwpf.usermodel.Range;
public class ConvertToMultiPageDoc {
public static void main(String[] args) throws Exception {
List<String> filepaths = new ArrayList<>();
filepaths.add("example1.txt");
filepaths.add("example2.txt");
String fileName = "example.doc";
// Create a new Word document
HWPFDocument document = new HWPFDocument();
Range range = document.getRange();
// Insert the content of each file into the document
for (int i = 0; i < filepaths.size(); i++) {
String filepath = filepaths.get(i);
String content = "Page " + (i+1) + ": " + readFile(filepath) + "\n";
range.insertAfter(content);
if (i != filepaths.size() - 1) {
range.insertAfter("\n");
}
}
// Save the document to a file
FileOutputStream out = new FileOutputStream(new File(fileName));
document.write(out);
out.close();
}
private static String readFile(String filepath) throws Exception {
// Read the content of a file
// This is just a simplified example, in reality you would want to handle exceptions and buffering
return new String(java.nio.file.Files.readAllBytes(java.nio.file.Paths.get(filepath)));
}
}
```
这个示例中,我们使用了一个`readFile`方法来读取文件内容。注意,在实际应用中,你需要对读取文件的过程进行错误处理和缓冲。
阅读全文
相关推荐
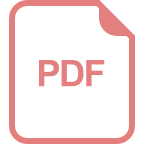
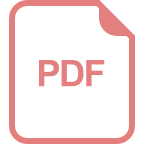















