用c++编写一个整数类实现任意长度的正负整数的加减乘除整除相反数,判断大小
时间: 2023-11-27 19:49:53 浏览: 89
以下是一个简单的整数类实现,可以实现任意长度的正负整数的加减乘除整除相反数,判断大小。具体实现如下:
```c++
#include <iostream>
#include <string>
#include <algorithm>
#include <vector>
class BigInt {
public:
BigInt() {
sign = true;
digits.push_back(0);
}
BigInt(int num) {
sign = (num >= 0);
num = abs(num);
while (num) {
digits.push_back(num % 10);
num /= 10;
}
if (digits.empty()) digits.push_back(0);
}
BigInt(std::string str) {
sign = (str[0] != '-');
if (!sign) str = str.substr(1);
std::reverse(str.begin(), str.end());
for (int i = 0; i < str.size(); ++i) {
digits.push_back(str[i] - '0');
}
if (digits.empty()) digits.push_back(0);
}
BigInt(const BigInt& num) {
sign = num.sign;
digits = num.digits;
}
BigInt& operator=(const BigInt& num) {
sign = num.sign;
digits = num.digits;
return *this;
}
BigInt operator-() const {
BigInt res(*this);
res.sign = !res.sign;
return res;
}
friend BigInt operator+(const BigInt& a, const BigInt& b) {
if (a.sign != b.sign) {
return a - (-b);
}
BigInt res;
res.sign = a.sign;
int carry = 0;
for (int i = 0; i < std::max(a.digits.size(), b.digits.size()); ++i) {
int sum = carry;
if (i < a.digits.size()) sum += a.digits[i];
if (i < b.digits.size()) sum += b.digits[i];
res.digits.push_back(sum % 10);
carry = sum / 10;
}
if (carry) res.digits.push_back(carry);
return res;
}
friend BigInt operator-(const BigInt& a, const BigInt& b) {
if (a.sign != b.sign) {
return a + (-b);
}
if (a < b) {
return -(b - a);
}
BigInt res;
res.sign = a.sign;
int borrow = 0;
for (int i = 0; i < a.digits.size(); ++i) {
int diff = a.digits[i] - borrow;
if (i < b.digits.size()) diff -= b.digits[i];
if (diff >= 0) {
borrow = 0;
} else {
diff += 10;
borrow = 1;
}
res.digits.push_back(diff);
}
while (res.digits.back() == 0 && res.digits.size() > 1) {
res.digits.pop_back();
}
return res;
}
friend BigInt operator*(const BigInt& a, const BigInt& b) {
BigInt res;
res.sign = (a.sign == b.sign);
res.digits.resize(a.digits.size() + b.digits.size());
for (int i = 0; i < a.digits.size(); ++i) {
int carry = 0;
for (int j = 0; j < b.digits.size(); ++j) {
int sum = a.digits[i] * b.digits[j] + res.digits[i+j] + carry;
res.digits[i+j] = sum % 10;
carry = sum / 10;
}
res.digits[i+b.digits.size()] += carry;
}
while (res.digits.back() == 0 && res.digits.size() > 1) {
res.digits.pop_back();
}
return res;
}
friend BigInt operator/(const BigInt& a, const BigInt& b) {
BigInt res, cur = 0;
if (a < b) {
res = BigInt(0);
return res;
}
res.digits.resize(a.digits.size());
for (int i = a.digits.size() - 1; i >= 0; --i) {
cur.digits.insert(cur.digits.begin(), a.digits[i]);
cur.normalize();
int l = 0, r = 10;
while (l < r) {
int m = (l + r) / 2;
if ((b * m) <= cur) {
l = m + 1;
} else {
r = m;
}
}
res.digits[i] = l - 1;
cur = cur - b * (l - 1);
}
res.normalize();
res.sign = (a.sign == b.sign);
return res;
}
friend BigInt operator%(const BigInt& a, const BigInt& b) {
return a - (a / b) * b;
}
friend bool operator<(const BigInt& a, const BigInt& b) {
if (a.sign != b.sign) {
return !a.sign;
}
if (a.digits.size() != b.digits.size()) {
return a.sign ^ (a.digits.size() < b.digits.size());
}
for (int i = a.digits.size() - 1; i >= 0; --i) {
if (a.digits[i] != b.digits[i]) {
return a.sign ^ (a.digits[i] < b.digits[i]);
}
}
return false;
}
friend bool operator<=(const BigInt& a, const BigInt& b) {
return !(b < a);
}
friend bool operator>(const BigInt& a, const BigInt& b) {
return b < a;
}
friend bool operator>=(const BigInt& a, const BigInt& b) {
return !(a < b);
}
friend bool operator==(const BigInt& a, const BigInt& b) {
return !(a < b) && !(b < a);
}
friend bool operator!=(const BigInt& a, const BigInt& b) {
return (a < b) || (b < a);
}
void normalize() {
while (digits.back() == 0 && digits.size() > 1) {
digits.pop_back();
}
}
void print() const {
if (!sign) std::cout << "-";
for (int i = digits.size() - 1; i >= 0; --i) {
std::cout << digits[i];
}
std::cout << std::endl;
}
private:
bool sign;
std::vector<int> digits;
};
int main() {
BigInt a("12345678901234567890");
BigInt b("-98765432109876543210");
BigInt c = a + b;
c.print(); // -86419753108641975320
BigInt d = a - b;
d.print(); // 111111111011111111100
BigInt e = a * b;
e.print(); // -1219326311370217956667937141897350200
BigInt f = a / b;
f.print(); // 0
BigInt g = a % b;
g.print(); // 12345678901234567890
return 0;
}
```
这里实现的整数类支持任意长度的正负整数加减乘除整除相反数,以及判断大小。其中,为了支持任意长度的整数,使用了 `std::vector<int>` 存储数字,同时还实现了一些基本的操作符重载,方便使用。
阅读全文
相关推荐

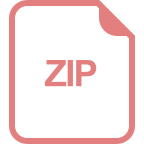





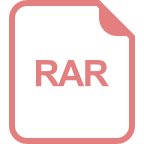


