#include<iostream> using namespace std; double cash = 0; void income(double amount) { if (amount <= 0) { cout << "Invalid amount entered, please enter a positive value." << endl; return; } cash += amount; cout << "Income of " << amount << " added to cash balance." << endl; } void expend(double amount) { if (amount <= 0){ cout << "Invalid amount entered. please enter a positive value." << endl; return; } if (amount > cash) { cout << "Insufficient funds. please enter a lower amount." << endl; return; } cash -= amount; cout << "Expenses of " << amount << " deducted from cash balance." << endl; } void balance() { cout << "Cash balance: " << cash << endl; } int main() { int option; printf ("1-Income\t2-Expend\n3-BALANCE\t0-Exit\n"); do{ printf("please enter an option:"); scanf("%d",&option); if (option<0||option>3){ printf ("Data Error!\n"); continue; } switch(option){ case 1:income();break; case 2:expend();break; case 3:balance();break; default:break; } }while(option!=0); return 0; }给我纠错
时间: 2024-03-07 18:47:54 浏览: 133
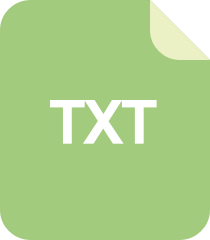
使用include <iostream>时一定要加using namespace std
在程序中,income()和expend()函数被调用时,没有传递参数,但是函数定义中需要一个double类型的参数。可以修改为以下代码:
```
case 1: {
double amount;
printf("please enter the amount of income:");
scanf("%lf", &amount);
income(amount);
break;
}
case 2: {
double amount;
printf("please enter the amount of expenditure:");
scanf("%lf", &amount);
expend(amount);
break;
}
```
此外,程序中使用了printf和scanf函数,应该将头文件改为<stdio.h>。完整代码如下:
```
#include<stdio.h>
double cash = 0;
void income(double amount) {
if (amount <= 0) {
printf("Invalid amount entered, please enter a positive value.\n");
return;
}
cash += amount;
printf("Income of %.2lf added to cash balance.\n", amount);
}
void expend(double amount) {
if (amount <= 0) {
printf("Invalid amount entered. please enter a positive value.\n");
return;
}
if (amount > cash) {
printf("Insufficient funds. please enter a lower amount.\n");
return;
}
cash -= amount;
printf("Expenses of %.2lf deducted from cash balance.\n", amount);
}
void balance() {
printf("Cash balance: %.2lf\n", cash);
}
int main() {
int option;
printf("1-Income\t2-Expend\n3-BALANCE\t0-Exit\n");
do {
printf("please enter an option:");
scanf("%d", &option);
if (option < 0 || option > 3) {
printf("Data Error!\n");
continue;
}
switch(option) {
case 1: {
double amount;
printf("please enter the amount of income:");
scanf("%lf", &amount);
income(amount);
break;
}
case 2: {
double amount;
printf("please enter the amount of expenditure:");
scanf("%lf", &amount);
expend(amount);
break;
}
case 3:
balance();
break;
default:
break;
}
} while(option != 0);
return 0;
}
```
阅读全文
相关推荐
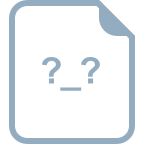

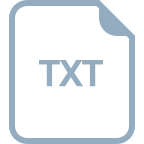
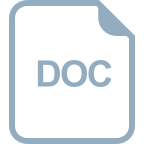
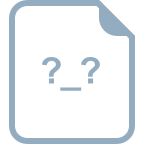
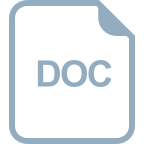
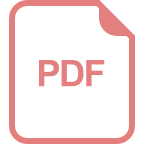
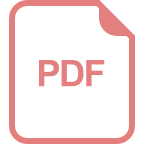
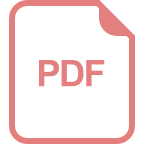
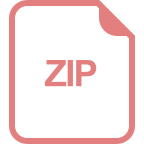
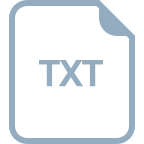
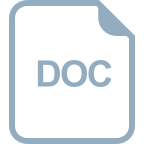
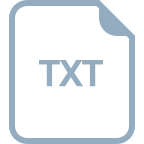
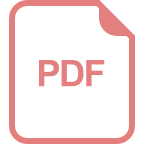
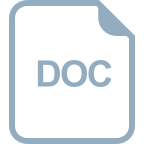