javafx tableview中赋值单元格
时间: 2023-08-24 21:06:12 浏览: 55
可以通过实现TableCell的updateItem()方法来赋值单元格,示例代码如下:
```java
public class MyTableCell extends TableCell<MyObject, String> {
@Override
protected void updateItem(String item, boolean empty) {
super.updateItem(item, empty);
if (empty || item == null) {
setText(null);
} else {
setText(item);
}
}
}
```
在TableView中设置对应列的cellFactory为MyTableCell即可。
相关问题
javafx tableview 给一列单元格赋值
可以使用setCellValueFactory()方法来给单元格赋值,具体实现可以参考以下代码:
TableColumn column = new TableColumn("姓名");
column.setCellValueFactory(new PropertyValueFactory<>("name"));
其中,"name"是你在数据模型中定义的属性名称。你可以在这个方法中指定需要使用哪个属性来赋值到表格中的单元格。
javaFx TableView
JavaFX TableView是一个可用于显示和编辑表格数据的UI组件。它是JavaFX框架中的一部分,提供了一种直观的方式来呈现数据,并允许用户进行排序、筛选和编辑。
要使用JavaFX TableView,你需要创建一个TableView对象,并将其添加到你的JavaFX应用程序的场景图中。然后,你需要定义表格的列(TableColumn),并将它们添加到TableView中。每个TableColumn都代表了表格中的一列,你可以指定列的标题、数据类型和渲染方式。
一旦你的TableView和TableColumn都设置好了,你需要创建一个ObservableList对象并将其作为数据源设置给TableView。ObservableList是JavaFX框架提供的一种特殊类型的列表,它可以自动监测数据的变化,并在数据改变时更新TableView的显示。
除了显示数据,JavaFX TableView还提供了一些内置的功能,如排序、筛选和编辑。你可以通过调用TableView的相应方法来启用这些功能,并在需要时处理相应的事件。
总之,JavaFX TableView是一个功能强大且易于使用的UI组件,适用于显示和编辑表格数据。它提供了丰富的功能和灵活的配置选项,可以满足各种不同的需求。
相关推荐
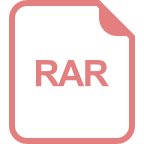












