请写出java实现ftp大文件多线程分段下载代码示例
时间: 2023-11-26 15:06:16 浏览: 73
以下是Java实现FTP大文件多线程分段下载的代码示例:
import java.io.*;
import java.net.*;
import java.util.concurrent.*;
public class FTPDownload {
private static final int NUM_THREADS = 10;
public static void main(String[] args) {
String ftpServer = "ftp.example.com";
String ftpFilePath = "/path/to/file";
String localFilePath = "/path/to/local/file";
ExecutorService executor = Executors.newFixedThreadPool(NUM_THREADS);
try {
URL url = new URL("ftp://" + ftpServer + ftpFilePath);
URLConnection connection = url.openConnection();
long fileSize = connection.getContentLengthLong();
RandomAccessFile localFile = new RandomAccessFile(localFilePath, "rw");
localFile.setLength(fileSize);
localFile.close();
long chunkSize = fileSize / NUM_THREADS;
long remainder = fileSize % NUM_THREADS;
CountDownLatch latch = new CountDownLatch(NUM_THREADS);
for (int i = 0; i < NUM_THREADS; i++) {
long startByte = i * chunkSize;
long endByte = (i == NUM_THREADS - 1) ? fileSize - 1 : startByte + chunkSize - 1;
executor.execute(new DownloadTask(ftpServer, ftpFilePath, localFilePath, startByte, endByte, latch));
}
latch.await();
} catch (Exception e) {
e.printStackTrace();
} finally {
executor.shutdown();
}
}
private static class DownloadTask implements Runnable {
private String ftpServer;
private String ftpFilePath;
private String localFilePath;
private long startByte;
private long endByte;
private CountDownLatch latch;
public DownloadTask(String ftpServer, String ftpFilePath, String localFilePath, long startByte, long endByte, CountDownLatch latch) {
this.ftpServer = ftpServer;
this.ftpFilePath = ftpFilePath;
this.localFilePath = localFilePath;
this.startByte = startByte;
this.endByte = endByte;
this.latch = latch;
}
public void run() {
try {
URL url = new URL("ftp://" + ftpServer + ftpFilePath);
URLConnection connection = url.openConnection();
connection.setRequestProperty("Range", "bytes=" + startByte + "-" + endByte);
InputStream input = connection.getInputStream();
RandomAccessFile localFile = new RandomAccessFile(localFilePath, "rw");
localFile.seek(startByte);
byte[] buffer = new byte[1024];
int bytesRead = 0;
while ((bytesRead = input.read(buffer)) != -1) {
localFile.write(buffer, 0, bytesRead);
}
input.close();
localFile.close();
latch.countDown();
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
笑话:为什么程序员总是说计算机只能做0和1的事情?因为他们从来都没有试过按下esc键!
阅读全文
相关推荐

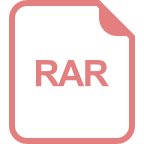













