python 怎么调用训练好的ssd模型
时间: 2023-07-10 20:31:23 浏览: 201
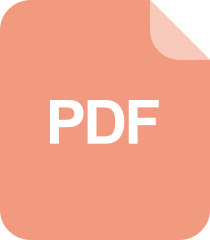
python 用opencv调用训练好的模型进行识别的方法
要调用训练好的SSD模型,可以使用TensorFlow提供的`tf.keras.models.load_model()`函数加载模型,并使用该模型进行推理。
以下是一个示例代码,可以加载一个预训练的SSD模型,并使用该模型进行对象检测:
```python
import tensorflow as tf
import cv2
import numpy as np
# 加载模型
model = tf.keras.models.load_model('path/to/your/ssd_model.h5')
# 加载标签
with open('path/to/your/label_map.txt', 'r') as f:
label_map = [line.strip() for line in f.readlines()]
# 加载图像
image = cv2.imread('path/to/your/image.jpg')
# 预处理图像
image = cv2.resize(image, (300, 300))
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
image = image / 255.0
# 进行推理
predictions = model.predict(np.array([image]))
# 处理预测结果
boxes = predictions[0][:, :4]
scores = predictions[0][:, 4:]
classes = np.argmax(scores, axis=1)
scores = np.max(scores, axis=1)
# 显示检测结果
for box, cls, score in zip(boxes, classes, scores):
if score > 0.5:
x1, y1, x2, y2 = box
label = label_map[cls]
cv2.rectangle(image, (int(x1 * 300), int(y1 * 300)), (int(x2 * 300), int(y2 * 300)), (0, 0, 255), 2)
cv2.putText(image, f'{label}: {score:.2f}', (int(x1 * 300), int(y1 * 300)), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 255), 1)
cv2.imshow('image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上述代码中,我们首先使用`tf.keras.models.load_model()`函数加载一个预训练的SSD模型。然后,我们加载标签,并使用OpenCV库加载图像。接下来,我们将图像缩放到300x300,并将像素值缩放到0到1之间。然后,我们使用模型进行推理,并处理预测结果。最后,我们在图像上绘制检测框并显示图像。
需要注意的是,上述代码中的路径需要根据实际情况进行修改。特别是,`ssd_model.h5`是一个预训练的SSD模型文件,`label_map.txt`是一个包含标签的文本文件。在使用上述代码之前,请确保已经下载并配置好了这些文件。
阅读全文
相关推荐
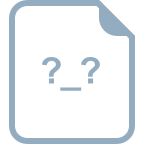
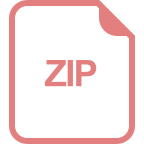
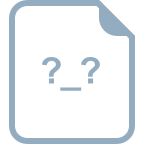
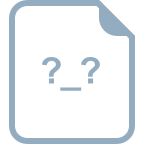
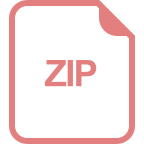
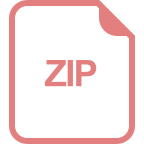
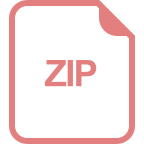
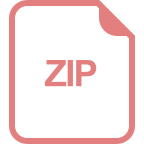
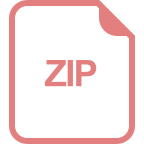
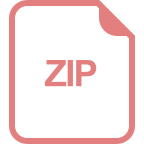
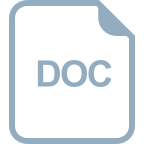
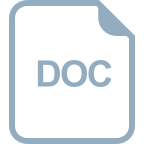
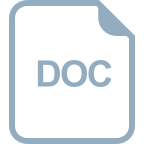
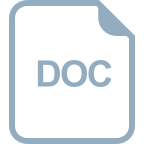
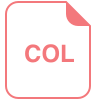
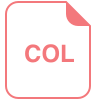
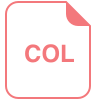
