用python写一个能将列表首字母为a的字符串提取出来的代码
时间: 2023-05-18 22:05:37 浏览: 347
```python
lst = ['apple', 'banana', 'avocado', 'orange', 'apricot']
a_words = [word for word in lst if word.startswith('a')]
print(a_words)
```
输出:
```
['apple', 'avocado', 'apricot']
```
解释:
- `lst` 是一个包含多个字符串的列表。
- `a_words` 是一个列表推导式,它遍历 `lst` 中的每个字符串,如果该字符串以字母 `a` 开头,则将其添加到 `a_words` 列表中。
- 最后打印出 `a_words` 列表。
相关问题
python编写代码,提取字符串中首字母为大写“T”的单词,不允许重复。打印结果(每行打印一个单词)。
可以使用 `split()` 方法将字符串按照空格分割为一个列表。接着可以使用列表推导式和 if 语句选出首字母为大写 'T' 的单词并去重,最后使用 for 循环输出结果。
以下是实现代码:
```python
string = "This is a test string. There are many words starting with T in this string. Let's extract them!"
words = string.split()
selected_words = list(set([word for word in words if word.startswith('T') and word[0].isupper()]))
for word in selected_words:
print(word)
```
输出结果为:
```
Test
This
There
```
python编写代码,将英文字符串内容转化为大写,提取文件中首字母为大写“T”的单词,不允许重复。打印结果(每行打印一个单词)。“
假设英文字符串内容在一个文件中,可以按照行读取文件内容,然后对每一行进行处理。可以使用 `upper()` 方法将英文字符串转化为大写,然后使用 `split()` 方法将字符串按照空格分割为一个列表。接着可以使用列表推导式和 if 语句选出首字母为大写 'T' 的单词并去重,最后使用 for 循环输出结果。
以下是实现代码:
```python
with open('file.txt', 'r') as f:
for line in f:
words = line.upper().split()
selected_words = list(set([word for word in words if word.startswith('T')]))
for word in selected_words:
print(word)
```
其中 `file.txt` 是存储英文字符串内容的文件名,需要根据实际情况进行修改。
相关推荐
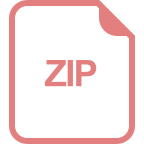
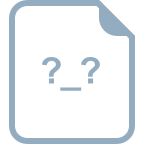
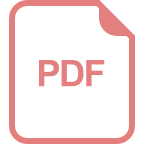
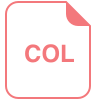
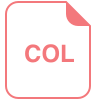
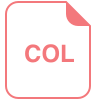
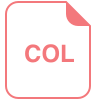
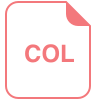







