Qt cpp 已知 可以通过调用 long long queryID = m_localPlayback->FPB_GetRecordTimeSegment 给服务端发消息要求查询录像时间,服务端会将消息返回值通过 SDK CallBack传出, 如何优雅的实现 批量调用m_localPlayback->FPB_GetRecordTimeSegment。获取数据,并等到所有结果都得到或者超时后,调用线程才继续执行。
时间: 2024-03-06 07:50:10 浏览: 17
可以使用 Qt 提供的 QFuture 和 QFutureWatcher 类来实现批量调用 `m_localPlayback->FPB_GetRecordTimeSegment` 并等待所有结果返回或者超时后继续执行。
具体步骤如下:
1. 定义一个 `QFutureWatcher` 对象和一个 `QList<QFuture<long long>>` 来存储异步操作的结果:
```cpp
QFutureWatcher<long long> watcher;
QList<QFuture<long long>> futures;
```
2. 在需要进行批量调用的地方,循环调用 `m_localPlayback->FPB_GetRecordTimeSegment` 并添加到 `futures` 列表中:
```cpp
for (int i = 0; i < count; i++) {
QFutureInterface<long long> *futureInterface = new QFutureInterface<long long>();
QtConcurrent::run([=]() {
long long result = m_localPlayback->FPB_GetRecordTimeSegment(arg1, arg2);
futureInterface->reportResult(result);
futureInterface->reportFinished();
});
futures.append(futureInterface->future());
}
```
这里使用 `QFutureInterface` 来手动创建异步操作,并将操作的结果交给 `QFutureInterface`,以便可以将异步操作的结果添加到 `futures` 列表中。
3. 连接 `QFutureWatcher` 的 `finished` 信号,以便在所有异步操作完成后处理结果:
```cpp
QObject::connect(&watcher, &QFutureWatcher<long long>::finished, [=]() {
QList<long long> results;
for (int i = 0; i < futures.count(); i++) {
QFuture<long long> future = futures.at(i);
if (future.isFinished() && !future.isCanceled()) {
results.append(future.result());
} else {
results.append(-1);
}
}
// 处理结果
});
```
4. 启动异步操作并等待结果,可以使用 `QFutureWatcher` 的 `setFuture` 函数来启动异步操作,并设置超时时间,以确保等待的时间不会过长:
```cpp
watcher.setFuture(QtConcurrent::mapped(futures, [](const QFuture<long long>& future) {
return future.result();
}));
if (!watcher.waitForFinished(timeout)) {
// 超时处理
}
```
这里使用了 `QtConcurrent::mapped` 函数来将 `QList<QFuture<long long>>` 转换为 `QFuture<QList<long long>>`,以便可以使用 `QFutureWatcher` 来监控所有异步操作的状态和结果。
完整的示例代码如下:
```cpp
QFutureWatcher<long long> watcher;
QList<QFuture<long long>> futures;
for (int i = 0; i < count; i++) {
QFutureInterface<long long> *futureInterface = new QFutureInterface<long long>();
QtConcurrent::run([=]() {
long long result = m_localPlayback->FPB_GetRecordTimeSegment(arg1, arg2);
futureInterface->reportResult(result);
futureInterface->reportFinished();
});
futures.append(futureInterface->future());
}
QObject::connect(&watcher, &QFutureWatcher<long long>::finished, [=]() {
QList<long long> results;
for (int i = 0; i < futures.count(); i++) {
QFuture<long long> future = futures.at(i);
if (future.isFinished() && !future.isCanceled()) {
results.append(future.result());
} else {
results.append(-1);
}
}
// 处理结果
});
watcher.setFuture(QtConcurrent::mapped(futures, [](const QFuture<long long>& future) {
return future.result();
}));
if (!watcher.waitForFinished(timeout)) {
// 超时处理
}
```
相关推荐








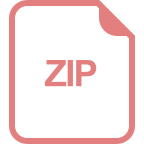
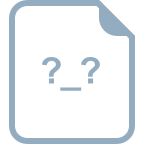