vue2中使用v-html解析后如何找到img文字进行判断呢
时间: 2024-03-17 13:41:31 浏览: 49
要找到 `v-html` 解析后的文本中的 `img` 标签,可以使用以下方法:
1. 在 `mounted` 钩子函数中获取 `v-html` 渲染后的元素,并使用 DOM 操作获取所有的 `img` 标签。
2. 使用 `querySelectorAll` 方法获取所有的 `img` 标签,然后遍历这些标签,获取 `alt` 属性的值进行判断。
3. 如果需要在 `v-html` 渲染完成后进行判断,可以使用 `nextTick` 方法等待 DOM 更新完成后再进行操作。
以下是一个示例代码:
```html
<template>
<div v-html="htmlStr"></div>
</template>
<script>
export default {
data() {
return {
htmlStr: '<p>这是一段包含<img src="xxx" alt="图片描述">图片的文本</p>'
}
},
mounted() {
const imgList = this.$el.querySelectorAll('img')
imgList.forEach(img => {
if (img.alt === '图片描述') {
console.log('找到了图片描述为"图片描述"的图片')
}
})
}
}
</script>
```
相关问题
vue2在el-table中v-html 解析后中找到文本中带有img 标签
在 `el-table` 中使用 `v-html` 渲染后,可以通过在 `cell-class-name` 属性中自定义样式,然后通过 `render` 函数获取当前单元格中的 `img` 标签,并进行判断。
具体实现步骤如下:
1. 在 `el-table` 中使用 `cell-class-name` 属性,自定义单元格的样式类名。
2. 在 `render` 函数中,获取当前单元格中的 `img` 标签,并进行判断。
以下是一个示例代码:
```html
<template>
<el-table :data="tableData" style="width: 100%">
<el-table-column prop="content" label="内容" :cell-class-name="getCellClassName">
</el-table-column>
</el-table>
</template>
<script>
export default {
data() {
return {
tableData: [
{
content: '<p>这是一段包含<img src="xxx" alt="图片描述">图片的文本</p>'
}
]
}
},
methods: {
getCellClassName({ row, column, rowIndex, columnIndex }) {
return 'my-cell-class'
}
},
render: function(h, { row, column, rowIndex, columnIndex }) {
if (columnIndex === 0) {
const htmlStr = row[column.property]
const div = document.createElement('div')
div.innerHTML = htmlStr
const imgList = div.querySelectorAll('img')
imgList.forEach(img => {
if (img.alt === '图片描述') {
// 图片描述为"图片描述"的处理逻辑
}
})
return h('div', htmlStr)
}
}
}
</script>
<style>
.my-cell-class {
padding: 0 !important; /* 去除单元格内边距 */
}
.my-cell-class > div {
padding: 10px; /* 设置单元格内边距 */
}
</style>
```
在上面的代码中,我们在 `getCellClassName` 方法中返回了自定义的样式类名 `my-cell-class`,并在样式中设置了 `padding: 0 !important` 去除单元格内边距,然后在 `render` 函数中获取当前单元格的 `htmlStr`,并使用 `querySelectorAll` 方法获取其中的 `img` 标签,进行判断。最后,使用 `h` 函数生成一个新的 `div` 元素,并将 `htmlStr` 作为其子元素返回。
vue使用v-html解析数据后如何添加v-model绑定
使用 v-html 指令渲染 HTML 是不支持将数据绑定到 HTML 元素上的,因为 v-html 只是将一个字符串插入到指定元素中,它本身并不是一个组件或元素。如果需要实现类似的功能,可以使用 Vue.js 提供的自定义组件来实现。
下面是一个示例代码,其中通过自定义组件 `v-html-input` 来实现将数据绑定到输入框中:
```html
<template>
<div>
<div v-html="html"></div>
<v-html-input v-model="html"></v-html-input>
</div>
</template>
<script>
Vue.component('v-html-input', {
template: '<input v-bind="$attrs" v-on="$listeners" v-model="innerHtml">',
props: ['value'],
computed: {
innerHtml: {
get: function () {
return this.value;
},
set: function (newValue) {
this.$emit('input', newValue);
}
}
}
});
new Vue({
el: '#app',
data: {
html: '<p>Hello, world!</p>'
}
});
</script>
```
在上面的示例中,我们通过自定义组件 `v-html-input` 来实现将数据绑定到输入框中。这个组件接收一个 value 属性,用来接收父组件传递的数据,同时使用 v-model 指令将输入框的值与内部的 innerHtml 属性双向绑定。当输入框的值发生变化时,通过 $emit 方法将变化的值发送一个 input 事件到父组件中,从而实现数据的双向绑定。
阅读全文
相关推荐
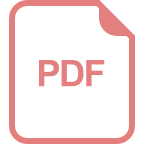
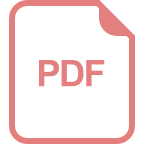
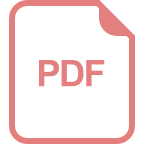













