c语言词法分析器代码
时间: 2023-08-01 15:12:31 浏览: 109
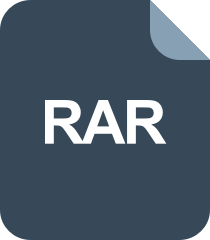
C语言词法分析器的代码
好的,以下是一个简单的C语言词法分析器代码示例:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
#define MAXLEN 100
#define MAXTOKENS 100
enum { NAME, PARENS, BRACKETS };
void dcl(void);
void dirdcl(void);
int gettoken(void);
int tokentype; // 最后一个记号的类型
char token[MAXTOKENS]; // 最后一个记号字符串
char name[MAXTOKENS]; // 标识符名
char datatype[MAXTOKENS]; // 数据类型为 char、int 等
char out[MAXLEN]; // 输出字符串
int main()
{
while (gettoken() != EOF) { // 直到文件结尾
strcpy(datatype, token); // 是一个类型说明符
out[0] = '\0';
dcl(); // 分析剩余部分
if (tokentype != '\n') {
printf("syntax error\n");
}
printf("%s: %s %s\n", name, out, datatype);
}
return 0;
}
// 返回下一个标记
int gettoken(void)
{
int c;
char *p = token;
while ((c = getchar()) == ' ' || c == '\t')
;
if (c == '(') {
if ((c = getchar()) == ')') {
strcpy(token, "()");
return tokentype = PARENS;
} else {
ungetc(c, stdin);
return tokentype = '(';
}
} else if (c == '[') {
for (*p++ = c; (*p++ = getchar()) != ']'; )
;
*p = '\0';
return tokentype = BRACKETS;
} else if (isalpha(c)) {
for (*p++ = c; isalnum(c = getchar()); )
*p++ = c;
*p = '\0';
ungetc(c, stdin);
return tokentype = NAME;
} else {
return tokentype = c;
}
}
// 分析一个声明
void dcl(void)
{
int ns;
for (ns = 0; gettoken() == '*'; ) // 统计字符 * 的个数
ns++;
dirdcl();
while (ns-- > 0) {
strcat(out, " pointer to");
}
}
// 分析一个直接声明
void dirdcl(void)
{
int type;
if (tokentype == '(') { // 形式为 ( dcl )
dcl();
if (tokentype != ')') {
printf("error: missing )\n");
}
} else if (tokentype == NAME) { // 变量名
strcpy(name, token);
} else {
printf("error: expected name or (dcl)\n");
}
while ((type = gettoken()) == PARENS || type == BRACKETS) {
if (type == PARENS) {
strcat(out, " function returning");
} else {
strcat(out, " array");
strcat(out, token);
strcat(out, " of");
}
}
}
```
这是一个简单的命令行程序,它从标准输入读取C语言声明,分析它们并将它们输出到标准输出。你可以根据自己的需要进行修改和扩展。
阅读全文
相关推荐
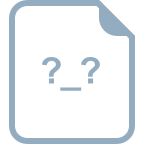
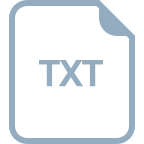
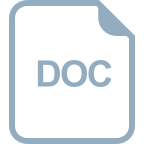

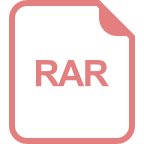
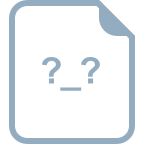
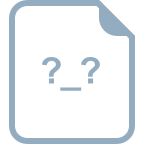
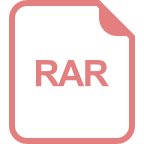
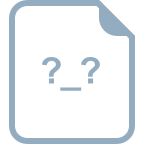
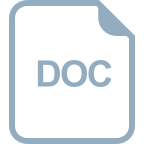
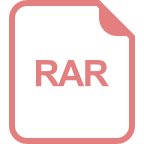
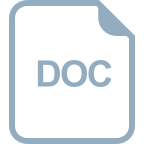
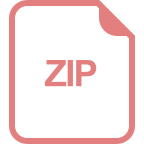