`tape` is required when a `Tensor` loss is passed.
时间: 2023-11-21 12:09:40 浏览: 39
The `tape` refers to the GradientTape in TensorFlow, which is used to compute gradients of a computation with respect to its input variables. When a `Tensor` loss is passed to a training function in TensorFlow, the gradients of the loss with respect to the trainable variables in the model need to be computed using the GradientTape.
Here is an example of how to use the GradientTape to compute gradients:
```python
import tensorflow as tf
# Define a simple model
model = tf.keras.Sequential([
tf.keras.layers.Dense(10, input_shape=(1,), activation='relu'),
tf.keras.layers.Dense(1)
])
# Define a loss function
loss_fn = tf.keras.losses.MeanSquaredError()
# Generate some dummy data
x = tf.random.normal((10, 1))
y = tf.random.normal((10, 1))
# Define an optimizer
optimizer = tf.keras.optimizers.SGD(learning_rate=1e-3)
# Train the model for one step
with tf.GradientTape() as tape:
# Compute the predictions of the model
predictions = model(x)
# Compute the loss between the predictions and the true values
loss = loss_fn(y, predictions)
# Compute the gradients of the loss with respect to the trainable variables
gradients = tape.gradient(loss, model.trainable_variables)
# Apply the gradients to the variables using the optimizer
optimizer.apply_gradients(zip(gradients, model.trainable_variables))
```
In this example, we define a simple model with one input variable and one output variable. We generate some dummy data and define a mean squared error loss function. We also define a stochastic gradient descent optimizer with a learning rate of 1e-3.
In the training loop, we use a GradientTape to record the operations performed by the model when computing the predictions and the loss. We then compute the gradients of the loss with respect to the trainable variables using the tape, and apply them to the variables using the optimizer.
相关推荐
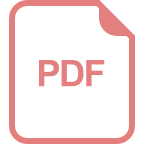
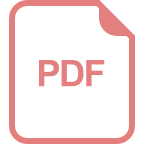
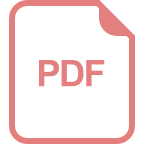





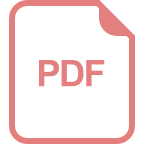
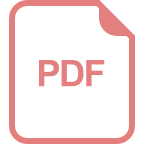
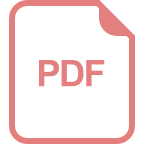
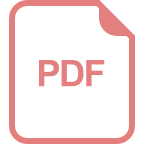
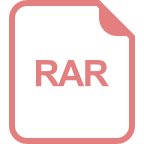
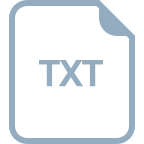