现有hi python hello python编写程序统计单词个数
时间: 2023-07-01 19:22:47 浏览: 51
可以使用Python中的字典来实现单词计数。具体代码如下:
```python
text = "hi python hello python"
words = text.split()
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
print(word_count)
```
输出结果为:
```
{'hi': 1, 'python': 2, 'hello': 1}
```
其中,首先使用 `split()` 方法将文本分割成单词列表,然后遍历单词列表,使用字典 `word_count` 记录每个单词出现的次数,最后输出字典即可。
相关问题
如何用python编写图形界面应用程序
Python提供了多种GUI库可以用来编写图形界面应用程序,常用的有Tkinter、PyQt、wxPython等。
以下是一个使用Tkinter库编写一个简单的图形界面应用程序的示例:
```python
import tkinter as tk
class Application(tk.Frame):
def __init__(self, master=None):
super().__init__(master)
self.master = master
self.pack()
self.create_widgets()
def create_widgets(self):
self.hi_there = tk.Button(self)
self.hi_there["text"] = "Hello World\n(click me)"
self.hi_there["command"] = self.say_hi
self.hi_there.pack(side="top")
self.quit = tk.Button(self, text="QUIT", fg="red",
command=self.master.destroy)
self.quit.pack(side="bottom")
def say_hi(self):
print("hi there, everyone!")
root = tk.Tk()
app = Application(master=root)
app.mainloop()
```
在这个示例中,我们创建了一个继承自`tkinter.Frame`的`Application`类,并在其中定义了一个`create_widgets`方法来创建界面元素。在`create_widgets`方法中,我们创建了一个按钮和一个退出按钮,并将它们放置在界面上。
当用户点击“Hello World”按钮时,`say_hi`方法会被调用,并打印一条消息。
最后,我们创建了一个`Tk`对象,将其作为`master`参数传递给`Application`对象,然后调用`mainloop`方法来启动应用程序的主循环。
需要注意的是,在实际应用程序中,需要根据需求自行设计和布局界面元素,并编写相应的事件处理方法。
用python 写一个界面程序
可以使用 Python 的 Tkinter 模块来编写界面程序。以下是一个简单的示例代码:
```python
import tkinter as tk
class Application(tk.Frame):
def __init__(self, master=None):
super().__init__(master)
self.master = master
self.pack()
self.create_widgets()
def create_widgets(self):
self.hi_there = tk.Button(self)
self.hi_there["text"] = "Hello World\n(click me)"
self.hi_there["command"] = self.say_hi
self.hi_there.pack(side="top")
self.quit = tk.Button(self, text="QUIT", fg="red",
command=self.master.destroy)
self.quit.pack(side="bottom")
def say_hi(self):
print("Hi there, everyone!")
root = tk.Tk()
app = Application(master=root)
app.mainloop()
```
这个程序创建了一个窗口,其中包含一个按钮和一个退出按钮。当用户点击“Hello World”按钮时,程序会在控制台输出一条消息。
相关推荐
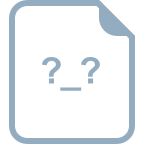
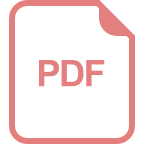
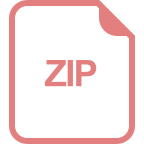












