实现未实现的 #ifndef __MYSTACK__ #define __MYSTACK__ #include <queue> using namespace std; class mystack { private: queue<int> q1; queue<int> q2; public: mystack(); void push(int); int pop(); int top(); bool empty(); }; #endif #include <iostream> #include <string> #include <queue> #include "mystack.h" using namespace std; void print(mystack& st) { while (!st.empty()) { int tmp = st.pop(); cout << tmp << ' '; } cout << endl; } int main() { mystack st; int n; cin >> n; while (n--) { string op; cin >> op; if (op == "push") { int x; cin >> x; st.push(x); cout << "push " << x << endl; } else if (op == "pop") { int res = st.pop(); if (res == -1) { cout << "pop failed" << endl; } else { cout << "pop " << res << endl; } } else if (op == "top") { int res = st.top(); if (res == -1) { cout << "top failed" << endl; } else { cout << "top " << res << endl; } } else if (op == "empty") { if (st.empty()) { cout << "st empty" << endl; } else { cout << "st not empty" << endl; } } } print(st); return 0; }
时间: 2024-03-17 09:44:07 浏览: 60
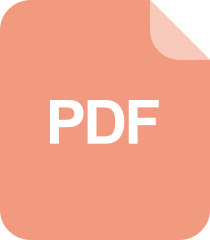
C++ 中pragma once 与 #ifndef _XXX_H_ #define _XXX_H_的区别

这是一个使用两个队列实现栈的程序,其中队列q1用于存储栈中的元素,而队列q2主要用于在push操作时辅助完成元素的入栈。
以下是完整的实现代码:
```cpp
#ifndef __MYSTACK__
#define __MYSTACK__
#include <queue>
using namespace std;
class mystack {
private:
queue<int> q1;
queue<int> q2;
public:
mystack() {}
void push(int x) {
q2.push(x);
while (!q1.empty()) {
q2.push(q1.front());
q1.pop();
}
swap(q1, q2);
}
int pop() {
if (q1.empty()) {
return -1;
}
int res = q1.front();
q1.pop();
return res;
}
int top() {
if (q1.empty()) {
return -1;
}
return q1.front();
}
bool empty() {
return q1.empty();
}
};
#endif
```
在主函数中,读入操作指令和操作数,然后调用mystack类的对应函数完成操作,并输出相应的结果。最后调用print函数输出栈中所有元素。
```cpp
#include <iostream>
#include <string>
#include "mystack.h"
using namespace std;
void print(mystack& st) {
while (!st.empty()) {
int tmp = st.pop();
cout << tmp << ' ';
}
cout << endl;
}
int main() {
mystack st;
int n;
cin >> n;
while (n--) {
string op;
cin >> op;
if (op == "push") {
int x;
cin >> x;
st.push(x);
cout << "push " << x << endl;
} else if (op == "pop") {
int res = st.pop();
if (res == -1) {
cout << "pop failed" << endl;
} else {
cout << "pop " << res << endl;
}
} else if (op == "top") {
int res = st.top();
if (res == -1) {
cout << "top failed" << endl;
} else {
cout << "top " << res << endl;
}
} else if (op == "empty") {
if (st.empty()) {
cout << "st empty" << endl;
} else {
cout << "st not empty" << endl;
}
}
}
print(st);
return 0;
}
```
阅读全文
相关推荐
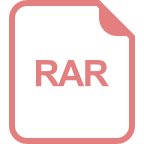
















