7-7 sdut-oop-8 分数四则运算(类和对象)
时间: 2023-05-31 12:18:38 浏览: 676
### 回答1:
这是一道关于分数四则运算的题目,需要使用面向对象编程的思想来解决。具体来说,需要定义一个分数类,包含分子和分母两个属性,以及加、减、乘、除四个方法,用于实现分数的四则运算。在进行运算时,需要先将两个分数化为通分,然后再进行相应的运算,最后将结果化简为最简分数。
### 回答2:
在一个分数类中,我们需要定义分数的四个基本运算:加、减、乘、除。这里我们可以使用类的方式来实现这些运算。
首先,我们要定义一个分数类,其中包含两个变量,分别表示分子和分母,同时也需要定义一个初始化函数用来初始化这两个变量。
在加法运算中,我们需要将两个分数相加,得到一个新的分数结果。因此,我们需要定义一个“+”函数,将两个分数对象作为参数传入,然后使用公式计算得到新分子和新分母。
class Fraction:
def __init__(self, numerator, denominator):
self.numerator = numerator
self.denominator = denominator
def __add__(self, other):
numerator = self.numerator*other.denominator + other.numerator*self.denominator
denominator = self.denominator*other.denominator
return Fraction(numerator, denominator)
在减法运算中,我们需要将两个分数相减,同样使用公式计算出新分子和新分母,然后返回一个新的分数对象。
def __sub__(self, other):
numerator = self.numerator*other.denominator - other.numerator*self.denominator
denominator = self.denominator*other.denominator
return Fraction(numerator, denominator)
在乘法运算中,我们需要将两个分数相乘,同样使用公式计算出新分子和新分母,然后返回一个新的分数对象。
def __mul__(self, other):
numerator = self.numerator*other.numerator
denominator = self.denominator*other.denominator
return Fraction(numerator, denominator)
在除法运算中,我们需要将两个分数相除,这里需要注意的是,当除数为0时,我们需要抛出一个异常,而当除数不为0时,同样使用公式计算出新分子和新分母,然后返回一个新的分数对象。
def __div__(self, other):
if other.numerator == 0:
raise ZeroDivisionError("division by zero")
numerator = self.numerator*other.denominator
denominator = self.denominator*other.numerator
return Fraction(numerator, denominator)
最后,我们可以创建两个分数对象,然后对它们进行四则运算。
fraction1 = Fraction(2, 3)
fraction2 = Fraction(1, 4)
result1 = fraction1 + fraction2
result2 = fraction1 - fraction2
result3 = fraction1 * fraction2
result4 = fraction1 / fraction2
print(result1.numerator, result1.denominator) # 11 12
print(result2.numerator, result2.denominator) # 5 12
print(result3.numerator, result3.denominator) # 1 6
print(result4.numerator, result4.denominator) # 8 3
### 回答3:
本题的主要难点在于实现分数的四则运算,并且要使用面向对象的方式。
首先,我们可以定义一个Fraction类,其中包括分子和分母两个属性。在该类中,我们需要定义以下方法:
1. __init__方法,用于初始化分数。
2. __str__方法,用于将分数以字符串的形式输出。
3. __add__方法,用于实现分数的加法。
4. __sub__方法,用于实现分数的减法。
5. __mul__方法,用于实现分数的乘法。
6. __truediv__方法,用于实现分数的除法。
具体实现如下:
```python
class Fraction:
def __init__(self, numerator, denominator):
self.numerator = numerator
self.denominator = denominator
def __str__(self):
return "%d/%d" % (self.numerator, self.denominator)
def __add__(self, other):
return Fraction(self.numerator * other.denominator + other.numerator * self.denominator,
self.denominator * other.denominator).simplify()
def __sub__(self, other):
return Fraction(self.numerator * other.denominator - other.numerator * self.denominator,
self.denominator * other.denominator).simplify()
def __mul__(self, other):
return Fraction(self.numerator * other.numerator, self.denominator * other.denominator).simplify()
def __truediv__(self, other):
return Fraction(self.numerator * other.denominator, self.denominator * other.numerator).simplify()
def simplify(self):
"""约分分数"""
if self.numerator == 0:
return Fraction(0, 1)
gcd = self._gcd(self.numerator, self.denominator)
return Fraction(self.numerator // gcd, self.denominator // gcd)
def _gcd(self, a, b):
"""计算最大公约数"""
if a % b == 0:
return b
else:
return self._gcd(b, a % b)
```
上述代码中,我们使用了__add__、__sub__、__mul__和__truediv__这些魔法方法来分别实现加法、减法、乘法和除法,使用了_simplify方法来约分分数。其中,__truediv__方法对应的是普通除法,而不是整除除法(符号为//)。此外,我们在构造函数__init__中初始化了分子和分母两个属性,并定义了一个__str__方法来将分数以字符串的形式输出。
通过上述代码,我们就可以对分数进行四则运算了。例如:
```python
# 5/6 + 3/4
f1 = Fraction(5, 6)
f2 = Fraction(3, 4)
print(f1 + f2) # 19/12
# 5/6 - 3/4
print(f1 - f2) # 1/12
# 5/6 * 3/4
print(f1 * f2) # 5/8
# 5/6 / 3/4
print(f1 / f2) # 10/9
```
最终,通过以上过程,我们已经成功实现了分数四则运算。
阅读全文
相关推荐
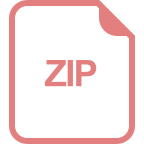
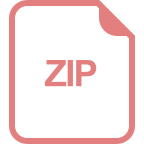















