flutter根据腾讯IM的SDK以及flutter插件flutter_map实现自定义消息发送位置信息,并以地图的形式展示在聊天框,用flutter代码实现
时间: 2024-06-08 21:10:57 浏览: 298
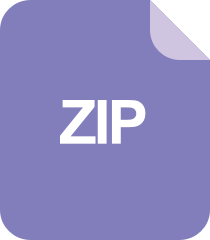
Flutter_slider_drawer:您可以通过此插件使滑块抽屉类型为ui
要实现这个功能,你需要先安装腾讯IM的Flutter插件`flutter_im_plugin`和flutter地图插件`flutter_map`。接下来,你需要按照以下步骤进行实现:
1. 引入插件包
在 `pubspec.yaml` 文件中引入以下两个插件:
```yaml
dependencies:
flutter:
sdk: flutter
flutter_im_plugin: ^1.1.2
flutter_map: ^0.13.1
```
2. 初始化腾讯IM SDK
在使用腾讯IM SDK前,你需要先初始化 SDK。在你的应用程序的启动页面或第一个页面,添加以下代码:
```dart
import 'package:flutter_im_plugin/flutter_im_plugin.dart';
void main() async {
// 初始化SDK
await FlutterImPlugin.init('你的SDKAppID');
runApp(MyApp());
}
```
3. 发送自定义消息
使用腾讯IM SDK的自定义消息,你可以构建并发送自己的消息。在本例中,我们将发送一个包含位置信息的自定义消息。
```dart
import 'package:flutter_im_plugin/flutter_im_plugin.dart';
void sendLocationMessage(String conversationId, double latitude, double longitude) {
// 构建自定义消息
final Map<String, dynamic> customMessage = {
'type': 'location',
'latitude': latitude,
'longitude': longitude,
};
// 发送自定义消息
FlutterImPlugin.sendMessage(
conversationId,
customMessage,
messageType: MessageType.Custom,
);
}
```
4. 监听收到的自定义消息
当收到自定义消息时,你需要解析消息并将其显示在地图上。在本例中,我们将使用 `flutter_map` 插件来显示位置信息。
```dart
import 'package:flutter_im_plugin/flutter_im_plugin.dart';
import 'package:flutter_map/flutter_map.dart';
import 'package:latlong/latlong.dart';
class MessageDetailPage extends StatefulWidget {
final Message message;
MessageDetailPage({Key key, this.message}) : super(key: key);
@override
_MessageDetailPageState createState() => _MessageDetailPageState();
}
class _MessageDetailPageState extends State<MessageDetailPage> {
MapController _mapController = MapController();
double _latitude;
double _longitude;
@override
void initState() {
super.initState();
// 解析自定义消息中的位置信息
final customMessage = widget.message.customMessage;
if (customMessage['type'] == 'location') {
_latitude = customMessage['latitude'];
_longitude = customMessage['longitude'];
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('位置信息'),
),
body: FlutterMap(
mapController: _mapController,
options: MapOptions(
center: LatLng(_latitude, _longitude),
zoom: 13,
),
layers: [
TileLayerOptions(
urlTemplate: 'https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png',
subdomains: ['a', 'b', 'c'],
),
MarkerLayerOptions(
markers: [
Marker(
width: 80.0,
height: 80.0,
point: LatLng(_latitude, _longitude),
builder: (ctx) => Container(
child: Icon(
Icons.location_on,
size: 50.0,
color: Colors.red,
),
),
),
],
),
],
),
);
}
}
```
在收到自定义消息时,你可以跳转到 `MessageDetailPage` 页面,并将消息对象传递给页面。在页面的 `initState` 方法中解析消息并在地图上显示位置信息。
以上就是使用腾讯IM SDK和 `flutter_map` 插件实现自定义消息发送位置信息并以地图形式展示在聊天框的示例代码。
阅读全文
相关推荐
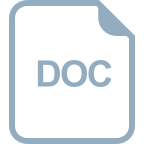
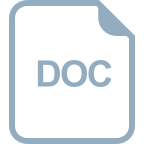
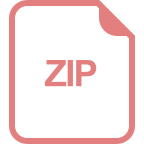
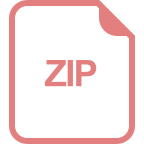
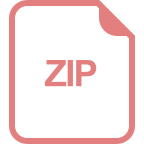
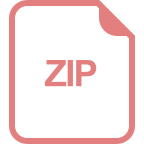
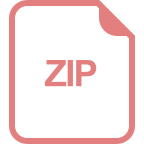
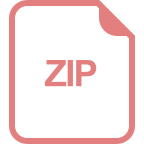
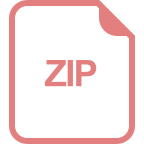
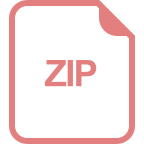
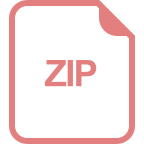
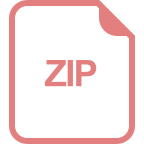
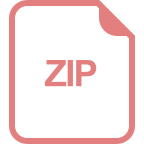
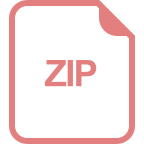
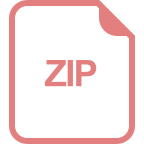
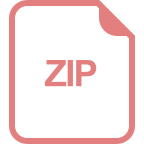
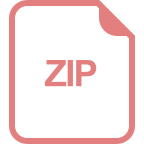
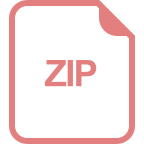