mybatisplus 如果根据时间自动创建表并插入数据
时间: 2023-07-26 18:05:50 浏览: 118
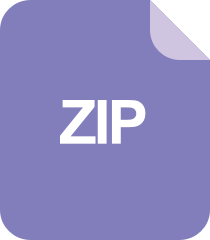
mybatisplus自动生成代
MybatisPlus本身不提供自动创建表并插入数据的功能,但可以通过编写代码实现该功能。具体实现步骤如下:
1. 定义需要自动创建表的实体类,并在实体类上添加@Table注解,指定表名和主键生成策略等信息。
2. 在实体类中定义需要创建的数据库表的字段。
3. 在MybatisPlus的配置文件中添加自动建表的配置项,如下:
```
mybatis-plus:
global-config:
db-config:
id-type: auto
table-prefix: mp_
# 自动建表
logic-delete-value: 1
logic-not-delete-value: 0
# 自动建表
auto-create-table: true
```
4. 在项目启动时,判断是否存在该表,如果不存在,则通过MybatisPlus的代码生成器生成表和实体类,并向表中插入数据。
```
// 判断表是否存在
boolean tableExist = false;
try {
Connection conn = dataSource.getConnection();
DatabaseMetaData metaData = conn.getMetaData();
ResultSet rs = metaData.getTables(null, null, tableName, null);
while (rs.next()) {
String name = rs.getString("TABLE_NAME");
if (tableName.equalsIgnoreCase(name)) {
tableExist = true;
break;
}
}
} catch (SQLException e) {
e.printStackTrace();
}
// 如果表不存在,则通过代码生成器生成表和实体类,并向表中插入数据
if (!tableExist) {
AutoGenerator mpg = new AutoGenerator();
// 配置数据源
mpg.setDataSource(dataSourceConfig);
// 包配置
mpg.setPackageInfo(new PackageConfig().setParent(packageName));
// 策略配置
mpg.setStrategy(new StrategyConfig()
.setNaming(NamingStrategy.underline_to_camel)
.setColumnNaming(NamingStrategy.underline_to_camel)
.setEntityLombokModel(true)
.setRestControllerStyle(true)
.setInclude(tableName)
.setControllerMappingHyphenStyle(true)
.setTablePrefix(tablePrefix)
);
// 执行生成代码
mpg.execute();
// 向表中插入数据
List<Entity> dataList = new ArrayList<>();
// 构造数据
...
// 批量插入数据
boolean insertResult = entityService.saveBatch(dataList);
}
```
需要注意的是,自动建表和数据插入的功能可能存在一些安全风险,因此在使用时需要谨慎考虑,避免出现意外情况。
阅读全文
相关推荐
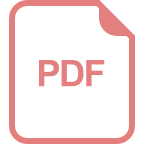
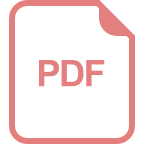
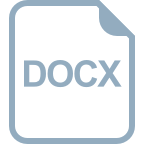
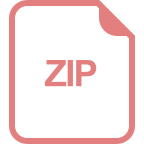
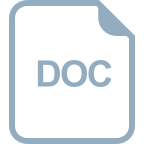
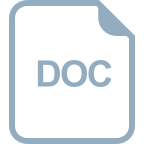










