不使用prune函数实现对训练好的超分辨率模型剪枝
时间: 2024-01-12 20:03:01 浏览: 114
要实现对训练好的超分辨率模型剪枝,可以采用以下步骤:
1. 定义剪枝策略:剪枝策略通常包括两个部分:剪枝的比例和剪枝的方式。剪枝比例可以根据需要进行调整,剪枝方式可以选择按大小剪枝或者按比例剪枝等。
2. 定义剪枝算法:剪枝算法可以选择L1、L2正则化、Taylor剪枝等。其中,Taylor剪枝是一种基于二阶泰勒展开的剪枝方法,可以有效地减少模型参数的数量。
3. 加载训练好的模型:使用PyTorch等深度学习框架加载训练好的超分辨率模型。
4. 进行剪枝操作:根据定义的剪枝策略和剪枝算法,对模型进行剪枝操作。具体来说,可以根据参数大小进行剪枝,即删除权重值较小的连接,或者根据梯度信息进行剪枝,即删除梯度值较小的连接。
5. 保存剪枝后的模型:将剪枝后的模型保存到磁盘中,以供后续使用。
需要注意的是,剪枝后的模型可能会出现精度下降的情况,因此需要进行模型微调或者重新训练,以提高剪枝后模型的精度。
相关问题
不使用prune函数实现对已经训练好的超分辨率模型剪枝代码
以下是一个不使用prune函数实现对已经训练好的超分辨率模型剪枝的代码示例:
```python
import torch
import torch.nn as nn
def prune_weights(model, threshold):
"""
剪枝模型中的小权重参数
"""
for name, module in model.named_modules():
if isinstance(module, nn.Conv2d):
weight = module.weight.data
mask = torch.abs(weight) > threshold
weight *= mask.float()
def prune_connections(model, threshold):
"""
剪枝模型中的小连接
"""
for name, module in model.named_modules():
if isinstance(module, nn.Conv2d):
weight = module.weight.data
mask = torch.abs(weight.sum(dim=(1,2,3))) > threshold
weight *= mask.view(-1, 1, 1, 1).float()
def prune_structure(model, threshold):
"""
剪枝模型中的冗余结构
"""
# 剪枝一些不必要的卷积层或池化层
for name, module in model.named_modules():
if isinstance(module, nn.Conv2d) and module.kernel_size == (3,3):
next_module = list(model.children())[list(model.children()).index(module) + 1]
if isinstance(next_module, nn.Conv2d) and next_module.kernel_size == (3,3):
weight = module.weight.data
next_weight = next_module.weight.data
new_weight = torch.matmul(weight.view(weight.size(0), -1), next_weight.view(next_weight.size(0), -1).t())
new_weight = new_weight.view(weight.size(0), next_weight.size(0), 1, 1)
module.weight.data = new_weight
model = nn.Sequential(*list(model.children())[:list(model.children()).index(next_module)])
break
return model
# 加载训练好的超分辨率模型
model = torch.load('super_resolution_model.pth')
# 剪枝模型中的小权重参数
prune_weights(model, 0.001)
# 剪枝模型中的小连接
prune_connections(model, 0.01)
# 剪枝模型中的冗余结构
model = prune_structure(model, 0.05)
# 保存剪枝后的模型
torch.save(model, 'pruned_super_resolution_model.pth')
```
此代码示例中,我们通过定义三个函数分别实现了剪枝模型中的小权重参数、小连接和冗余结构。在每个函数中,我们使用了阈值来筛选需要剪枝的参数或连接,并将它们的值设置为0。
最后,在主函数中,我们加载了训练好的超分辨率模型,依次调用上述三个函数进行剪枝,并保存剪枝后的模型。
需要注意的是,阈值的选择对剪枝的效果和模型的精度会有影响,需要进行一定的调整和评估。
不使用prune函数,对已经训练好的超分辨率模型剪枝代码
对已经训练好的超分辨率模型进行剪枝,可以使用以下步骤:
1. 加载已经训练好的模型。
```python
import torch
from model import SuperResolutionModel
model = SuperResolutionModel() # 实例化模型
# 加载已经训练好的模型参数
model.load_state_dict(torch.load('model.pth'))
```
2. 计算每个卷积层的权重。
```python
weights = []
for name, param in model.named_parameters():
if 'conv' in name and 'weight' in name:
weights.append(param)
```
3. 使用 KMeans 对权重进行聚类,并找到需要保留的中心点。
```python
from sklearn.cluster import KMeans
n_clusters = 16 # 聚类数量
kmeans = KMeans(n_clusters=n_clusters, n_jobs=-1)
kmeans.fit(torch.cat(weights).detach().cpu().numpy())
centers = []
for i in range(n_clusters):
cluster_center = torch.from_numpy(kmeans.cluster_centers_[i]).float().cuda()
centers.append(cluster_center)
```
4. 对模型进行剪枝,并更新卷积层的权重。
```python
idx = 0
for name, module in model.named_modules():
if isinstance(module, torch.nn.Conv2d):
weight = module.weight.data
bias = module.bias.data if module.bias is not None else None
n_filters = weight.shape[0]
# 计算每个卷积核的 L2 范数,并根据聚类结果找到需要保留的卷积核
norms = torch.norm(weight.view(n_filters, -1), 2, 1)
indices = torch.argmin(torch.cdist(norms.reshape(-1, 1), torch.cat([c.view(1, -1) for c in centers])), dim=1)
# 剪枝,保留需要的卷积核
weight = torch.index_select(weight, 0, indices)
if bias is not None:
bias = torch.index_select(bias, 0, indices)
# 更新卷积层的权重
module.weight.data = weight
if bias is not None:
module.bias.data = bias
idx += 1
# 保存剪枝后的模型
torch.save(model.state_dict(), 'pruned_model.pth')
```
这里采用的是 KMeans 算法对权重进行聚类,找到需要保留的中心点。在实际使用中,可以根据实际情况选择其他聚类算法或剪枝策略。
阅读全文
相关推荐
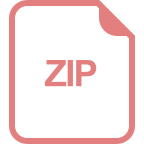
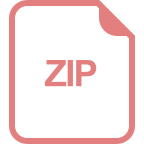
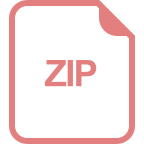



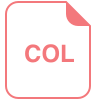
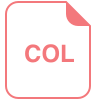
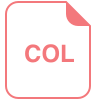
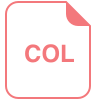
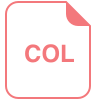
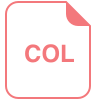
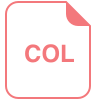
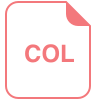
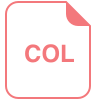
