单链表数据结构构建c语言
时间: 2023-08-24 18:05:00 浏览: 93
以下是单链表数据结构的 C 语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义单链表节点结构体
struct Node {
int data; // 存储节点的数据
struct Node* next; // 存储指向下一个节点的指针
};
// 在链表头部插入一个节点
void insertAtHead(struct Node** headRef, int newData) {
// 创建新节点
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = newData;
// 新节点指向当前头节点
newNode->next = *headRef;
// 更新头指针
*headRef = newNode;
}
// 在链表尾部插入一个节点
void insertAtTail(struct Node** headRef, int newData) {
// 创建新节点
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = newData;
newNode->next = NULL;
// 如果链表为空,则将新节点设置为头节点
if (*headRef == NULL) {
*headRef = newNode;
return;
}
// 遍历链表,找到最后一个节点
struct Node* lastNode = *headRef;
while (lastNode->next != NULL) {
lastNode = lastNode->next;
}
// 将新节点添加到最后一个节点后面
lastNode->next = newNode;
}
// 在链表中查找一个节点
struct Node* searchNode(struct Node* head, int searchData) {
// 遍历链表,查找节点
while (head != NULL) {
if (head->data == searchData) {
return head;
}
head = head->next;
}
// 如果没有找到,则返回 NULL
return NULL;
}
// 从链表中删除一个节点
void deleteNode(struct Node** headRef, int deleteData) {
// 如果链表为空,则直接返回
if (*headRef == NULL) {
return;
}
// 如果要删除的节点是头节点,则更新头指针
if ((*headRef)->data == deleteData) {
*headRef = (*headRef)->next;
return;
}
// 遍历链表,查找要删除的节点
struct Node* prevNode = *headRef;
struct Node* currNode = (*headRef)->next;
while (currNode != NULL) {
if (currNode->data == deleteData) {
// 将前一个节点的 next 指针指向当前节点的下一个节点
prevNode->next = currNode->next;
// 释放当前节点的内存空间
free(currNode);
return;
}
prevNode = currNode;
currNode = currNode->next;
}
}
// 打印链表
void printList(struct Node* head) {
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
int main() {
// 创建一个空链表
struct Node* head = NULL;
// 在链表头部插入节点
insertAtHead(&head, 5);
insertAtHead(&head, 4);
insertAtHead(&head, 3);
insertAtHead(&head, 2);
insertAtHead(&head, 1);
// 在链表尾部插入节点
insertAtTail(&head, 6);
insertAtTail(&head, 7);
insertAtTail(&head, 8);
// 打印链表
printList(head); // 输出:1 2 3 4 5 6 7 8
// 查找节点
struct Node* searchResult = searchNode(head, 3);
if (searchResult != NULL) {
printf("Found: %d\n", searchResult->data); // 输出:Found: 3
} else {
printf("Not found.\n");
}
// 删除节点
deleteNode(&head, 2);
// 打印链表
printList(head); // 输出:1 3 4 5 6 7 8
return 0;
}
```
以上代码实现了单链表的基本操作,包括在链表头部插入节点、在链表尾部插入节点、查找节点、删除节点等。
阅读全文
相关推荐
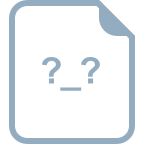
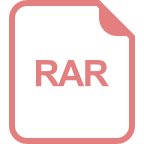
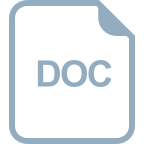
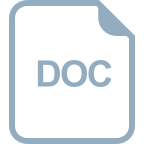
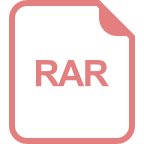
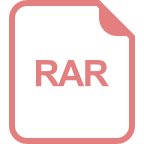
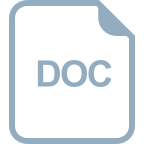
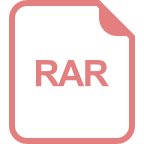
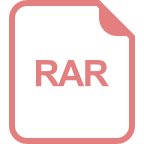
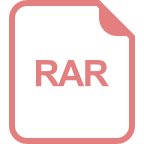
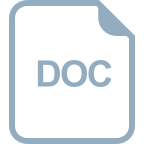
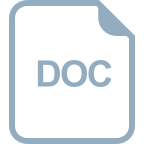
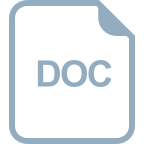

