springboot整合netty做聊天
时间: 2023-09-11 09:00:27 浏览: 254
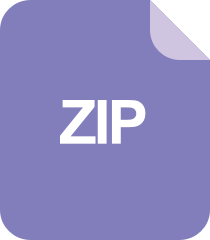
springboot与netty整合

### 回答1:
Spring Boot可以很方便地整合Netty来实现聊天功能。Netty是一个高性能的网络编程框架,可以用来实现各种网络应用,包括聊天室。
在Spring Boot中,可以使用Netty的ChannelHandler来处理客户端连接、消息接收和发送等操作。可以使用Spring Boot的WebSocket支持来实现浏览器与服务器之间的实时通信。
具体实现步骤如下:
1. 引入Netty和WebSocket的依赖
在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.25.Final</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-websocket</artifactId>
</dependency>
```
2. 创建Netty服务器
创建一个Netty服务器,监听指定的端口,处理客户端连接和消息接收等操作。可以使用Spring Boot的@Configuration注解和@Bean注解来创建Netty服务器。
```
@Configuration
public class NettyConfig {
@Value("${netty.port}")
private int port;
@Autowired
private ChannelInitializer<SocketChannel> channelInitializer;
@Bean
public EventLoopGroup bossGroup() {
return new NioEventLoopGroup();
}
@Bean
public EventLoopGroup workerGroup() {
return new NioEventLoopGroup();
}
@Bean
public ServerBootstrap serverBootstrap() {
ServerBootstrap serverBootstrap = new ServerBootstrap();
serverBootstrap.group(bossGroup(), workerGroup())
.channel(NioServerSocketChannel.class)
.childHandler(channelInitializer);
return serverBootstrap;
}
@Bean
public ChannelFuture channelFuture() throws InterruptedException {
return serverBootstrap().bind(port).sync();
}
}
```
其中,@Value("${netty.port}")注解用来读取配置文件中的端口号,@Autowired注解用来注入ChannelInitializer,用于处理客户端连接和消息接收等操作。
3. 创建ChannelInitializer
创建一个ChannelInitializer,用于初始化Netty的ChannelPipeline,添加ChannelHandler来处理客户端连接和消息接收等操作。
```
@Component
public class ChatServerInitializer extends ChannelInitializer<SocketChannel> {
@Autowired
private ChatServerHandler chatServerHandler;
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new HttpServerCodec());
pipeline.addLast(new HttpObjectAggregator(65536));
pipeline.addLast(new WebSocketServerProtocolHandler("/chat"));
pipeline.addLast(chatServerHandler);
}
}
```
其中,HttpServerCodec用于处理HTTP请求和响应,HttpObjectAggregator用于将HTTP请求和响应合并为一个完整的消息,WebSocketServerProtocolHandler用于处理WebSocket握手和消息传输,chatServerHandler用于处理客户端连接和消息接收等操作。
4. 创建ChannelHandler
创建一个ChannelHandler,用于处理客户端连接和消息接收等操作。
```
@Component
@ChannelHandler.Sharable
public class ChatServerHandler extends SimpleChannelInboundHandler<TextWebSocketFrame> {
private static final ChannelGroup channels = new DefaultChannelGroup(GlobalEventExecutor.INSTANCE);
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
channels.add(ctx.channel());
}
@Override
public void channelInactive(ChannelHandlerContext ctx) throws Exception {
channels.remove(ctx.channel());
}
@Override
protected void channelRead(ChannelHandlerContext ctx, TextWebSocketFrame msg) throws Exception {
String message = msg.text();
channels.writeAndFlush(new TextWebSocketFrame(message));
}
}
```
其中,@ChannelHandler.Sharable注解用于标记该ChannelHandler可以被多个Channel共享,channels用于保存所有连接的Channel,channelActive方法用于添加新的Channel,channelInactive方法用于移除已关闭的Channel,channelRead方法用于处理接收到的消息,将消息广播给所有连接的客户端。
5. 创建WebSocket客户端
在前端页面中创建WebSocket客户端,连接到Netty服务器,发送和接收消息。
```
var socket = new WebSocket("ws://localhost:808/chat");
socket.onopen = function(event) {
console.log("WebSocket connected.");
};
socket.onmessage = function(event) {
console.log("Received message: " + event.data);
};
socket.onclose = function(event) {
console.log("WebSocket closed.");
};
function sendMessage() {
var message = document.getElementById("message").value;
socket.send(message);
}
```
其中,WebSocket连接的URL为ws://localhost:808/chat,onopen方法用于在连接建立时输出日志,onmessage方法用于在接收到消息时输出日志,onclose方法用于在连接关闭时输出日志,sendMessage方法用于发送消息。
6. 运行程序
运行Spring Boot程序,访问前端页面,即可实现聊天功能。
以上就是使用Spring Boot整合Netty实现聊天功能的步骤。
### 回答2:
Spring Boot是一个用来简化创建Spring应用程序的框架,而Netty是一个高性能的网络编程框架。将它们整合起来可以实现一个简单的聊天应用程序。
首先,我们需要创建一个基于Spring Boot的项目。可以使用Spring Initializer工具来快速生成一个基本的Spring Boot项目骨架。在pom.xml文件中添加Netty的依赖,这样项目就可以使用Netty库。
接下来,创建一个Netty的服务器类,用于处理客户端的连接和消息。在这个类中,我们需要实现Netty的ChannelInboundHandlerAdapter接口来处理连接和消息的事件。在连接建立时,可以将连接信息保存到一个Map中,以便后续消息的转发。当接收到消息时,可以根据消息内容将消息转发给指定的客户端。
在Spring Boot的配置文件中,我们需要配置Netty服务器的监听端口和服务器线程池的大小等参数。可以使用Spring Boot的注解来标记需要进行自动配置的类和方法。
在Spring Boot的控制器中,我们可以添加一个用于接收用户发送的消息的API接口。当这个接口被调用时,它会将用户发送的消息转发给Netty服务器,然后由服务器进行处理和转发。
此外,可以根据实际需求添加一些其他的功能,比如用户身份验证、消息加解密等。可以在Netty服务器的代码中添加相应的逻辑来实现这些功能。
最后,我们可以使用Spring Boot的打包工具将项目打包成一个可执行的Jar文件。然后可以将这个Jar文件部署到服务器上,并启动它来运行这个聊天应用程序。
总之,通过将Spring Boot和Netty整合起来,我们可以快速构建一个简单的聊天应用程序。Spring Boot提供了便捷的开发和配置方式,而Netty能够提供高性能的网络通信能力,这使得我们可以很容易地实现一个高效的聊天系统。
阅读全文
相关推荐
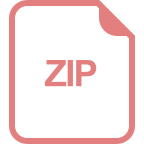
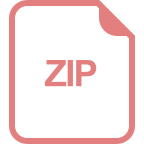
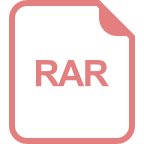
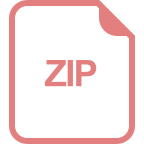
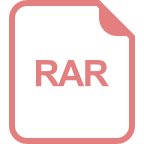
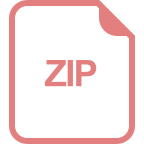
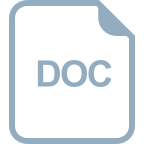
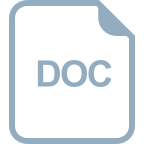
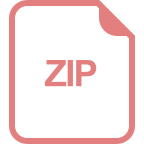
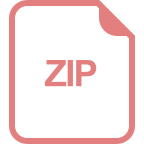
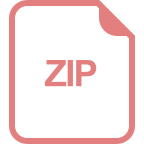
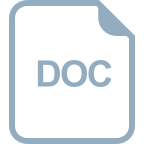
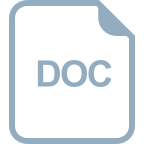
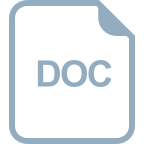
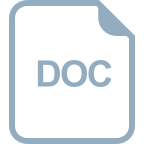