使用three.js生成铁路运行线路
时间: 2023-03-27 20:02:35 浏览: 311
我可以回答这个问题。使用three.js可以生成铁路运行线路,可以通过three.js提供的API来创建线条和曲线,然后将其添加到场景中。同时,可以使用three.js提供的材质和光源来渲染线路,使其看起来更加真实。
相关问题
前端如何使用Three.js 生成3D人物模型
要使用Three.js生成3D人物模型,需要掌握以下技能:
1. Three.js基础知识:包括场景、相机、渲染器、几何形状、材质、光源等基本概念。
2. 3D建模软件:需要使用3D建模软件(如Blender、Maya等)创建人物模型,并将其导出为可在Three.js中使用的格式(如OBJ、FBX等)。
3. Three.js加载器:需要使用Three.js中的加载器(如OBJLoader、FBXLoader等)将人物模型加载到Three.js中。
4. Three.js材质和光源:需要使用Three.js中的材质和光源来渲染人物模型,使其看起来更真实。
以下是使用Three.js生成3D人物模型的基本步骤:
1. 创建场景、相机和渲染器。
2. 使用3D建模软件创建人物模型,并将其导出为OBJ或FBX格式。
3. 使用Three.js中的加载器将人物模型加载到场景中。
4. 添加材质和光源来渲染人物模型。
5. 调整相机位置和角度以获得所需的视角和效果。
需要注意的是,生成3D人物模型是一个相对复杂的过程,需要耐心和技巧。同时,由于人物模型的复杂性和文件大小,可能需要进行优化和性能调整,以确保在网页上流畅地加载和呈现。
在vue2中使用three.js生成一个大型模型
在Vue2中使用Three.js生成一个大型模型需要以下步骤:
1. 安装Three.js
可以通过npm安装Three.js
```
npm install three
```
2. 在Vue组件中引入Three.js
在Vue组件中引入Three.js
```javascript
import * as THREE from 'three'
```
3. 创建一个Three.js场景
```javascript
mounted() {
// 创建场景
this.scene = new THREE.Scene();
// 创建相机
this.camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
// 创建渲染器
this.renderer = new THREE.WebGLRenderer();
this.renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(this.renderer.domElement);
}
```
4. 创建一个大型模型
```javascript
mounted() {
// 创建场景
this.scene = new THREE.Scene();
// 创建相机
this.camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
// 创建渲染器
this.renderer = new THREE.WebGLRenderer();
this.renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(this.renderer.domElement);
// 创建几何体
const geometry = new THREE.BoxGeometry(1, 1, 1);
// 创建材质
const material = new THREE.MeshBasicMaterial({
color: 0x00ff00
});
// 创建网格
this.cube = new THREE.Mesh(geometry, material);
// 将网格添加到场景中
this.scene.add(this.cube);
// 设置相机位置
this.camera.position.z = 5;
}
```
5. 渲染场景
```javascript
mounted() {
// 创建场景
this.scene = new THREE.Scene();
// 创建相机
this.camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
// 创建渲染器
this.renderer = new THREE.WebGLRenderer();
this.renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(this.renderer.domElement);
// 创建几何体
const geometry = new THREE.BoxGeometry(1, 1, 1);
// 创建材质
const material = new THREE.MeshBasicMaterial({
color: 0x00ff00
});
// 创建网格
this.cube = new THREE.Mesh(geometry, material);
// 将网格添加到场景中
this.scene.add(this.cube);
// 设置相机位置
this.camera.position.z = 5;
// 渲染场景
const animate = () => {
requestAnimationFrame(animate);
this.cube.rotation.x += 0.01;
this.cube.rotation.y += 0.01;
this.renderer.render(this.scene, this.camera);
};
animate();
}
```
以上就是在Vue2中使用Three.js生成一个大型模型的步骤。具体的实现可以根据需求进行调整。
阅读全文
相关推荐
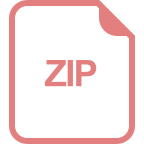
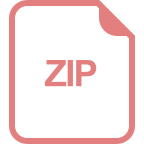
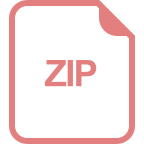
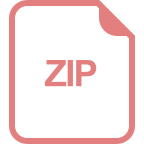
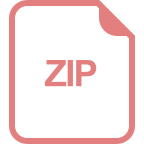
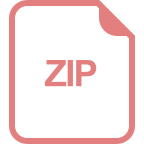
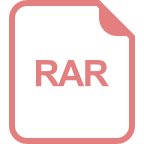
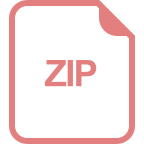
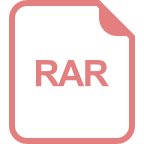



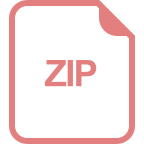
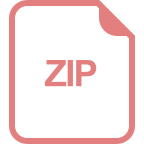
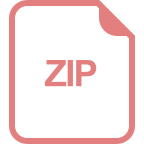