如何调用 //判断学生平均成绩的大小 bool operator>(Student &other){ return other.average_score() > average; } }; bool compare_average(Student &s1,Student &s2) { return s1.average_score() > s2.average_score();
时间: 2024-02-19 22:57:24 浏览: 29
你可以使用 `std::sort` 函数,结合函数指针或函数对象,对一个 `Student` 类型的数组进行排序。
如果你想使用函数指针来对数组进行排序,可以定义一个比较函数指针,然后把指针传递给 `std::sort` 函数。比较函数指针的参数类型应该与 `Student` 类型的数组元素相同,返回值类型应该是 `bool` 类型。下面是一个示例代码:
```c++
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
class Student {
public:
string name;
double score[3];
double average_score() {
return (score[0] + score[1] + score[2]) / 3.0;
}
// 判断学生平均成绩的大小
bool operator>(Student &other) {
return other.average_score() > average_score();
}
};
bool compare_average(Student &s1, Student &s2) {
return s1.average_score() > s2.average_score();
}
int main() {
// 声明一个 Student 类型的数组
Student students[] = {
{"Alice", {90, 85, 95}},
{"Bob", {80, 95, 75}},
{"Charlie", {85, 80, 90}}
};
int n = sizeof(students) / sizeof(students[0]);
// 使用函数指针进行排序
sort(students, students + n, compare_average);
// 输出排序结果
for(int i = 0; i < n; i++) {
cout << students[i].name << " " << students[i].average_score() << endl;
}
return 0;
}
```
上面的代码中,我们定义了一个 `compare_average` 函数,用于比较两个 `Student` 类型的元素的平均成绩大小,然后把这个函数的指针传递给 `std::sort` 函数,对 `students` 数组进行排序。最后输出排序结果。
如果你想使用函数对象来对数组进行排序,可以定义一个比较函数对象,并把函数对象传递给 `std::sort` 函数。下面是一个示例代码:
```c++
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
class Student {
public:
string name;
double score[3];
double average_score() {
return (score[0] + score[1] + score[2]) / 3.0;
}
// 判断学生平均成绩的大小
bool operator>(Student &other) {
return other.average_score() > average_score();
}
};
struct CompareAverage {
bool operator()(Student &s1, Student &s2) {
return s1.average_score() > s2.average_score();
}
};
int main() {
// 声明一个 Student 类型的数组
Student students[] = {
{"Alice", {90, 85, 95}},
{"Bob", {80, 95, 75}},
{"Charlie", {85, 80, 90}}
};
int n = sizeof(students) / sizeof(students[0]);
// 使用函数对象进行排序
sort(students, students + n, CompareAverage());
// 输出排序结果
for(int i = 0; i < n; i++) {
cout << students[i].name << " " << students[i].average_score() << endl;
}
return 0;
}
```
上面的代码中,我们定义了一个 `CompareAverage` 结构体,用于比较两个 `Student` 类型的元素的平均成绩大小,然后把这个结构体的对象传递给 `std::sort` 函数,对 `students` 数组进行排序。最后输出排序结果。
相关推荐
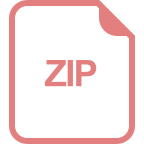










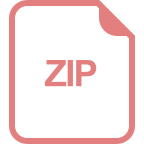
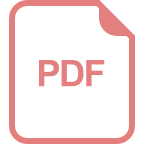
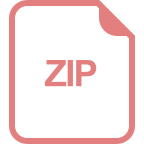
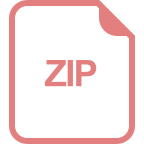