请给出上述思路的代码
时间: 2024-02-22 09:55:17 浏览: 16
以下是使用Python实现Christofides算法的示例代码:
```python
import numpy as np
import networkx as nx
import matplotlib.pyplot as plt
def tsp_artist(image_path):
# Step 1: Convert image to graph
img = plt.imread(image_path)
height, width, _ = img.shape
nodes = []
for i in range(height):
for j in range(width):
nodes.append((i, j))
G = nx.Graph()
for i in range(len(nodes)):
for j in range(i+1, len(nodes)):
dist = np.linalg.norm(np.array(nodes[i]) - np.array(nodes[j]))
G.add_edge(nodes[i], nodes[j], weight=dist)
# Step 2: Compute MST
T = nx.minimum_spanning_tree(G)
# Step 3: Find odd-degree vertices
odd_nodes = []
for node in T.nodes():
if T.degree(node) % 2 == 1:
odd_nodes.append(node)
# Step 4: Find minimum-weight perfect matching on odd-degree vertices
subgraph = G.subgraph(odd_nodes)
matching = nx.algorithms.matching.max_weight_matching(subgraph)
# Step 5: Add matching edges to MST
for edge in matching:
T.add_edge(*edge)
# Step 6: Find Eulerian circuit of the modified graph
circuit = list(nx.eulerian_circuit(T))
# Step 7: Remove repeated vertices to get Hamiltonian cycle
path = [circuit[0][0]]
for edge in circuit:
if edge[1] not in path:
path.append(edge[1])
path.append(path[0])
# Plot the Hamiltonian cycle on the image
plt.imshow(img)
plt.plot([p[1] for p in path], [p[0] for p in path], 'r')
plt.show()
# Example usage
tsp_artist('image.jpg')
```
注:这里使用了Python的networkx库来进行图论计算,matplotlib库来进行图像的可视化。
相关推荐
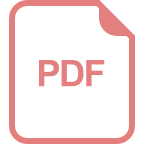
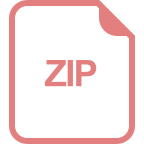
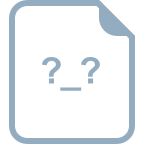
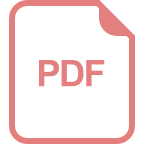
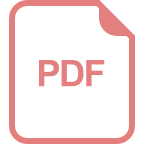
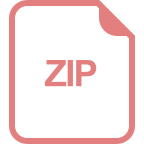
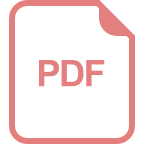
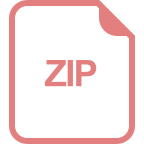
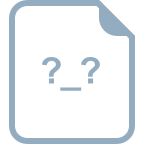
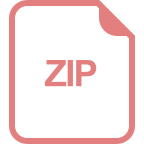
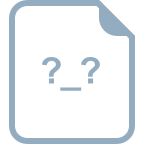
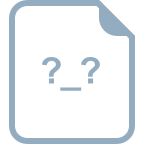
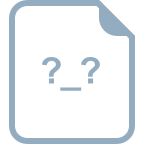
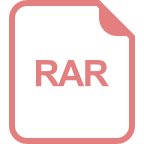
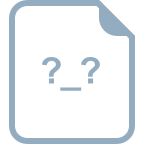
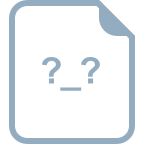
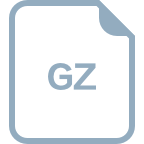