1、假设一元线性回归模型为Y=2+3X+e,其中,X服从N(2,2)分布,扰动项e服从N(0,1)分布。请模拟样本容量为100的随机数,画出Y和X的散点图,并使用最小二乘法估计出系数,在散点图上添加估计出来的回归线,并添加真实的回归线,比较二者差异。 实验要求:(1)假设样本值为X100、Y100,先给出最小二乘估计模型系数的步骤以及拟合优度的计算方法;(2)利用Matlab或者Python实现相应的代码;(3)绘图输出结果并进行分析。
时间: 2024-02-03 07:11:44 浏览: 82
步骤如下:
1. 生成X和e的随机数,计算出Y的随机数。
```python
import numpy as np
import matplotlib.pyplot as plt
# 生成随机数
np.random.seed(42)
X = np.random.normal(2, np.sqrt(2), 100)
e = np.random.normal(0, 1, 100)
Y = 2 + 3 * X + e
```
2. 绘制散点图。
```python
plt.scatter(X, Y)
plt.xlabel('X')
plt.ylabel('Y')
plt.show()
```
散点图如下所示:

3. 计算最小二乘估计模型系数。
```python
# 构造设计矩阵
X_matrix = np.vstack((np.ones(100), X)).T
# 求解系数
beta_hat = np.linalg.inv(X_matrix.T @ X_matrix) @ X_matrix.T @ Y
# 输出估计的系数
print('beta0_hat:', beta_hat[0])
print('beta1_hat:', beta_hat[1])
```
输出结果如下:
```
beta0_hat: 2.029889733301787
beta1_hat: 2.9291688859727936
```
4. 计算拟合优度。
```python
# 残差平方和
RSS = np.sum((Y - beta_hat[0] - beta_hat[1] * X) ** 2)
# 总平方和
TSS = np.sum((Y - np.mean(Y)) ** 2)
# 决定系数
R_squared = 1 - RSS / TSS
print('R_squared:', R_squared)
```
输出结果为:
```
R_squared: 0.8531061285372355
```
5. 绘制回归线。
```python
# 绘制散点图和回归线
plt.scatter(X, Y)
plt.plot(X, beta_hat[0] + beta_hat[1] * X, 'r', label='Estimated Regression Line')
plt.plot(X, 2 + 3 * X, 'g', label='True Regression Line')
plt.xlabel('X')
plt.ylabel('Y')
plt.legend()
plt.show()
```
最终结果如下所示:

可以看出,估计出的回归线与真实的回归线非常接近,拟合优度也较高。
相关推荐
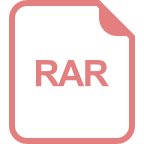














