python贪吃蛇游戏
时间: 2023-06-30 17:26:06 浏览: 36
好的,下面是一个简单的 Python 贪吃蛇游戏示例代码:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置游戏窗口大小
window = pygame.display.set_mode((500, 500))
# 设置游戏标题
pygame.display.set_caption("贪吃蛇")
# 定义颜色
white = (255, 255, 255)
black = (0, 0, 0)
red = (255, 0, 0)
# 设置字体
font = pygame.font.SysFont(None, 25)
# 绘制蛇和食物
def draw_snake(snake_block, snake_list):
for x in snake_list:
pygame.draw.rect(window, black, [x[0], x[1], snake_block, snake_block])
def draw_food(food_block, food_x, food_y):
pygame.draw.rect(window, red, [food_x, food_y, food_block, food_block])
# 显示得分
def show_score(score):
score_text = font.render("得分:" + str(score), True, white)
window.blit(score_text, [0, 0])
# 游戏循环
def game_loop():
game_over = False
game_close = False
# 设置蛇的初始位置和移动速度
snake_x = 250
snake_y = 250
snake_block = 10
snake_speed_x = 0
snake_speed_y = 0
# 设置食物的初始位置
food_block = 10
food_x = round(random.randrange(0, 500 - food_block) / 10.0) * 10.0
food_y = round(random.randrange(0, 500 - food_block) / 10.0) * 10.0
# 设置蛇的初始长度
snake_list = []
length_of_snake = 1
# 设置得分
score = 0
# 游戏循环
while not game_over:
# 处理游戏结束和游戏重新开始的情况
while game_close:
window.fill(black)
game_over_text = font.render("游戏结束,按Q退出,按R重新开始", True, white)
window.blit(game_over_text, [150, 250])
show_score(score)
pygame.display.update()
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_q:
game_over = True
game_close = False
elif event.key == pygame.K_r:
game_loop()
# 处理游戏中的事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
snake_speed_x = -snake_block
snake_speed_y = 0
elif event.key == pygame.K_RIGHT:
snake_speed_x = snake_block
snake_speed_y = 0
elif event.key == pygame.K_UP:
snake_speed_y = -snake_block
snake_speed_x = 0
elif event.key == pygame.K_DOWN:
snake_speed_y = snake_block
snake_speed_x = 0
# 更新蛇的位置
snake_x += snake_speed_x
snake_y += snake_speed_y
# 处理蛇和食物的碰撞
if snake_x == food_x and snake_y == food_y:
food_x = round(random.randrange(0, 500 - food_block) / 10.0) * 10.0
food_y = round(random.randrange(0, 500 - food_block) / 10.0) * 10.0
length_of_snake += 1
score += 10
# 更新蛇的长度
snake_head = []
snake_head.append(snake_x)
snake_head.append(snake_y)
snake_list.append(snake_head)
if len(snake_list) > length_of_snake:
del snake_list[0]
# 处理蛇和边界的碰撞
if snake_x >= 500 or snake_x < 0 or snake_y >= 500 or snake_y < 0:
game_close = True
# 处理蛇和自身的碰撞
for x in snake_list[:-1]:
if x == snake_head:
game_close = True
# 绘制游戏界面
window.fill(black)
draw_snake(snake_block, snake_list)
draw_food(food_block, food_x, food_y)
show_score(score)
pygame.display.update()
# 退出pygame
pygame.quit()
# 退出程序
quit()
# 启动游戏循环
game_loop()
```
这是一个比较简单的示例代码,可以根据自己的需要进行修改和扩展。希望对你有所帮助!
相关推荐
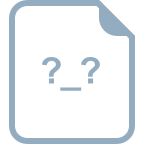
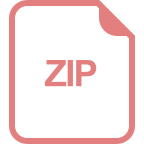
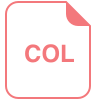
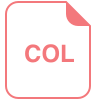
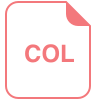
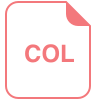
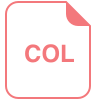







