GD32F130 串口收发
时间: 2023-07-23 21:22:59 浏览: 134
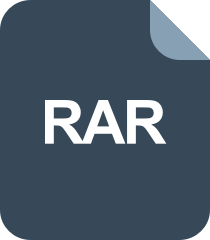
GD32芯片USB虚拟串口收发程序
下面是一个基本的GD32F130串口收发的代码示例,使用了GD32的标准库:
```
#include "gd32f1x0.h"
/* 定义串口收发缓存区 */
#define BUF_SIZE 64
uint8_t uart_receive_buf[BUF_SIZE];
uint8_t uart_send_buf[BUF_SIZE] = "Hello, world!\r\n";
/* 定义串口配置结构体 */
usart_parameter_struct uart_init_struct;
int main(void)
{
/* 使能串口时钟 */
rcu_periph_clock_enable(RCU_USART0);
/* 配置串口引脚 */
gpio_af_set(GPIOA, GPIO_AF_1, GPIO_PIN_2 | GPIO_PIN_3);
gpio_mode_set(GPIOA, GPIO_MODE_AF, GPIO_PUPD_NONE, GPIO_PIN_2 | GPIO_PIN_3);
gpio_output_options_set(GPIOA, GPIO_OTYPE_PP, GPIO_OSPEED_10MHZ, GPIO_PIN_2 | GPIO_PIN_3);
/* 配置串口参数 */
usart_deinit(USART0);
uart_init_struct.usart_baudrate = 115200;
uart_init_struct.usart_word_length = USART_WL_8BIT;
uart_init_struct.usart_stop_bits = USART_STB_1;
uart_init_struct.usart_parity = USART_PM_NONE;
uart_init_struct.usart_hardware_flow_control = USART_HFCTRL_NONE;
uart_init_struct.usart_mode = USART_MODE_TX_RX;
usart_init(USART0, &uart_init_struct);
/* 使能串口接收中断 */
usart_interrupt_enable(USART0, USART_INT_RBNE);
/* 使能串口 */
usart_enable(USART0);
while (1)
{
/* 发送数据 */
usart_data_transmit(USART0, uart_send_buf[0]);
}
}
/* 串口中断处理函数 */
void USART0_IRQHandler(void)
{
if (usart_interrupt_flag_get(USART0, USART_INT_FLAG_RBNE))
{
/* 接收数据 */
uint8_t ch = usart_data_receive(USART0);
/* 处理接收到的数据 */
}
}
```
这个代码中,我们使用GD32的标准库来进行串口的配置和收发。首先,我们使用rcu_periph_clock_enable函数使能USART0的时钟,并使用gpio_af_set和gpio_mode_set函数配置串口引脚。然后,我们使用usart_init函数配置串口参数,并使用usart_interrupt_enable函数使能串口接收中断。最后,我们在主循环中使用usart_data_transmit函数发送数据,在中断处理函数中使用usart_data_receive函数接收数据。
阅读全文
相关推荐
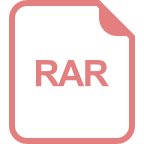
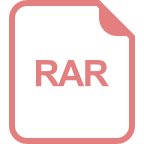
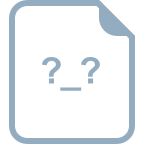
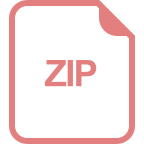
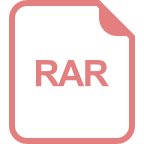
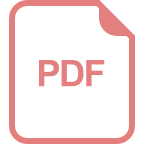
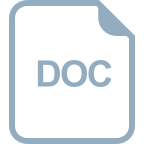
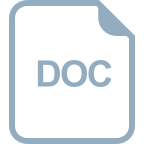
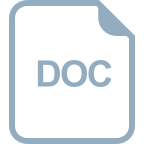
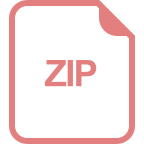
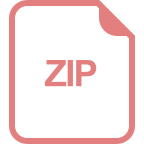
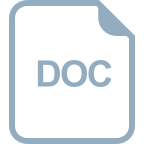
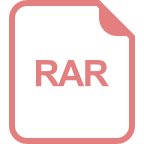
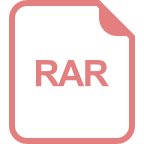
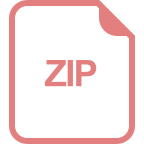
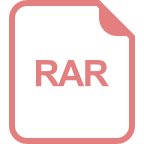
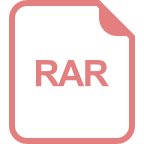