java 动态二维数组文件读写
时间: 2023-10-02 19:13:23 浏览: 47
1. 文件读取
```java
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class Dynamic2DArrayFileReader {
public static void main(String[] args) {
try {
File file = new File("input.txt");
Scanner scanner = new Scanner(file);
// Read the number of rows and columns
int rows = scanner.nextInt();
int cols = scanner.nextInt();
// Initialize the 2D array
int[][] array = new int[rows][cols];
// Read the elements of the array
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
array[i][j] = scanner.nextInt();
}
}
// Print the array
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
System.out.print(array[i][j] + " ");
}
System.out.println();
}
scanner.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
```
2. 文件写入
```java
import java.io.File;
import java.io.FileNotFoundException;
import java.io.PrintWriter;
public class Dynamic2DArrayFileWriter {
public static void main(String[] args) {
int[][] array = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
try {
File file = new File("output.txt");
PrintWriter writer = new PrintWriter(file);
// Write the number of rows and columns
writer.println(array.length);
writer.println(array[0].length);
// Write the elements of the array
for (int i = 0; i < array.length; i++) {
for (int j = 0; j < array[0].length; j++) {
writer.print(array[i][j] + " ");
}
writer.println();
}
writer.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
```
相关推荐
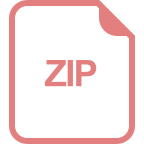
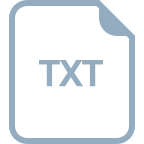
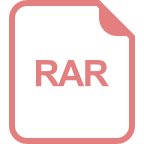










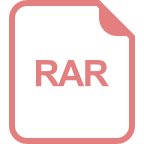
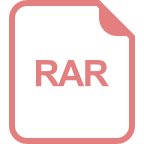
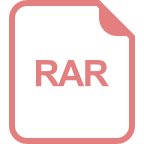
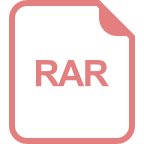
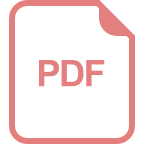
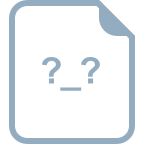