ubuntu环境下用c语言编写kittler阈值分割算法
时间: 2024-02-12 09:06:08 浏览: 100
在Ubuntu环境下使用C语言编写Kittler阈值分割算法的步骤如下:
1. 安装OpenCV库
在终端中输入以下命令:
```
sudo apt-get install libopencv-dev
```
2. 创建工程
在终端中创建一个新目录,并在该目录下创建一个名为`kittler_thresholding.c`的C文件:
```
mkdir kittler_thresholding
cd kittler_thresholding
touch kittler_thresholding.c
```
3. 编写代码
在`kittler_thresholding.c`文件中编写以下代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <opencv2/opencv.hpp>
using namespace cv;
int main(int argc, char** argv)
{
if (argc != 2)
{
printf("Usage: %s <image_path>\n", argv[0]);
return -1;
}
// 读取灰度图像
Mat img = imread(argv[1], IMREAD_GRAYSCALE);
// 计算直方图
int histSize = 256;
float range[] = { 0, 256 };
const float* histRange = { range };
Mat hist;
calcHist(&img, 1, 0, Mat(), hist, 1, &histSize, &histRange, true, false);
// 初始化最小方差为正无穷
double min_var = std::numeric_limits<double>::infinity();
int threshold = 0;
// 遍历所有阈值,计算方差
for (int t = 0; t < 256; t++)
{
// 计算类别1的权重与均值
double w1 = cv::sum(hist.rowRange(0, t+1))[0];
if (w1 == 0)
continue;
double mu1 = cv::sum(hist.rowRange(0, t+1).mul(cv::Mat::ones(1, t+1, CV_32F) * cv::Mat_<float>(range)))[0] / w1;
// 计算类别2的权重与均值
double w2 = cv::sum(hist.rowRange(t+1, histSize))[0];
if (w2 == 0)
continue;
double mu2 = cv::sum(hist.rowRange(t+1, histSize).mul(cv::Mat::ones(1, histSize-t-1, CV_32F) * cv::Mat_<float>(range+t+1)))[0] / w2;
// 计算类别1和类别2的方差之和
double var = w1 * w2 * pow(mu1 - mu2, 2);
// 更新最小方差和阈值
if (var < min_var)
{
min_var = var;
threshold = t;
}
}
// 二值化图像
Mat binary;
threshold(img, binary, threshold, 255, THRESH_BINARY);
// 显示结果
namedWindow("Original Image", WINDOW_AUTOSIZE);
imshow("Original Image", img);
namedWindow("Binary Image", WINDOW_AUTOSIZE);
imshow("Binary Image", binary);
waitKey(0);
return 0;
}
```
4. 编译和运行
在终端中输入以下命令编译程序:
```
g++ -o kittler_thresholding kittler_thresholding.c `pkg-config --cflags --libs opencv`
```
然后在终端中运行以下命令:
```
./kittler_thresholding image.jpg
```
程序会读取`image.jpg`图像并进行Kittler阈值分割,将结果显示出来。
以上是在Ubuntu环境下使用C语言编写Kittler阈值分割算法的步骤,希望对您有所帮助。
阅读全文
相关推荐
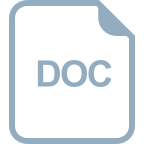
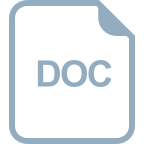
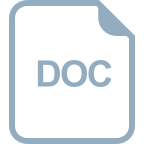

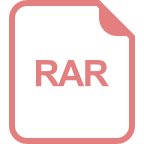
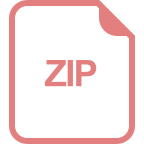

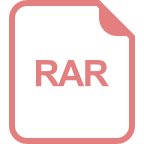
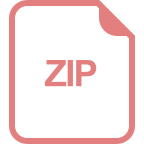
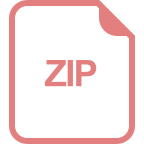
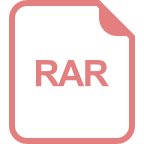
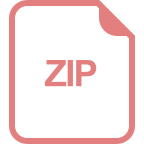
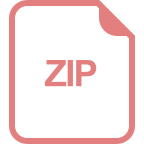
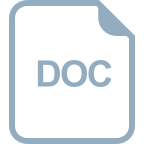
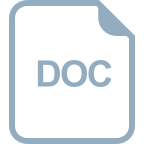
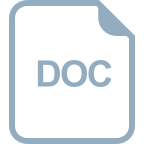
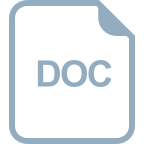
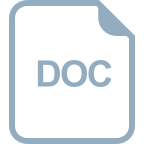
