C# vue多自定义组件表单生成pdf
时间: 2023-07-25 17:17:07 浏览: 181
要使用C#和Vue生成PDF,可以使用以下步骤:
1. 在Vue中使用多个自定义组件来构建表单,使用Vue的模板语法和指令来定义表单元素和验证规则。
2. 使用Vue的axios库将表单数据发送到后端C#应用程序。
3. 在C#中使用iTextSharp或其他PDF生成库将表单数据转换为PDF文件。
下面是一个简单的示例,演示了如何使用Vue和C#生成PDF:
在Vue中定义表单:
```
<template>
<form>
<custom-input v-model="name" label="Name"></custom-input>
<custom-input v-model="email" label="Email" type="email"></custom-input>
<custom-select v-model="gender" label="Gender" :options="['Male', 'Female']"></custom-select>
<custom-checkbox v-model="terms" label="I agree to the terms and conditions"></custom-checkbox>
<button @click.prevent="submitForm">Submit</button>
</form>
</template>
<script>
import axios from 'axios';
import CustomInput from './CustomInput.vue';
import CustomSelect from './CustomSelect.vue';
import CustomCheckbox from './CustomCheckbox.vue';
export default {
data() {
return {
name: '',
email: '',
gender: '',
terms: false
}
},
components: {
CustomInput,
CustomSelect,
CustomCheckbox
},
methods: {
submitForm() {
axios.post('/api/pdf', {
name: this.name,
email: this.email,
gender: this.gender,
terms: this.terms
})
.then(response => {
// handle PDF response
})
.catch(error => {
console.error(error);
});
}
}
}
</script>
```
在C#中处理表单数据并生成PDF:
```
using iTextSharp.text;
using iTextSharp.text.pdf;
using System.IO;
using System.Web.Http;
public class PdfController : ApiController
{
[HttpPost]
[Route("api/pdf")]
public HttpResponseMessage GeneratePdf([FromBody] FormData formData)
{
var document = new Document();
var output = new MemoryStream();
var writer = PdfWriter.GetInstance(document, output);
document.Open();
var font = FontFactory.GetFont(BaseFont.HELVETICA, BaseFont.CP1252, BaseFont.NOT_EMBEDDED);
document.Add(new Paragraph($"Name: {formData.Name}", font));
document.Add(new Paragraph($"Email: {formData.Email}", font));
document.Add(new Paragraph($"Gender: {formData.Gender}", font));
document.Add(new Paragraph($"Terms: {(formData.Terms ? "Yes" : "No")}", font));
document.Close();
var response = new HttpResponseMessage();
response.Content = new ByteArrayContent(output.ToArray());
response.Content.Headers.ContentDisposition = new System.Net.Http.Headers.ContentDispositionHeaderValue("attachment");
response.Content.Headers.ContentDisposition.FileName = "form.pdf";
response.Content.Headers.ContentType = new System.Net.Http.Headers.MediaTypeHeaderValue("application/pdf");
return response;
}
public class FormData
{
public string Name { get; set; }
public string Email { get; set; }
public string Gender { get; set; }
public bool Terms { get; set; }
}
}
```
在这个示例中,使用了iTextSharp库来生成PDF文件。在C#的控制器中,定义了一个名为`FormData`的类来表示表单数据。在`GeneratePdf`方法中,将表单数据添加到PDF文件中,并将PDF文件作为HTTP响应返回。在Vue中,使用axios库将表单数据发送到后端C#应用程序。当接收到PDF响应时,可以将其保存到本地或直接在浏览器中打开。
阅读全文
相关推荐
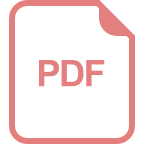
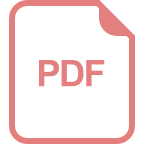















