java程序json-lib第三方库怎么将对象写到json文件中,如何读取并创建对象
时间: 2024-02-22 19:56:57 浏览: 18
要将一个Java对象写入JSON文件,你可以使用json-lib第三方库中的JSONObject类。以下是一个示例:
```java
import net.sf.json.JSONObject;
import java.io.FileWriter;
import java.io.IOException;
public class JsonExample {
public static void main(String[] args) {
// 创建一个Java对象
Person person = new Person("John", "Doe", 30);
// 将Java对象转换成JSONObject
JSONObject jsonObject = JSONObject.fromObject(person);
// 写入JSON文件
try {
FileWriter file = new FileWriter("person.json");
file.write(jsonObject.toString());
file.flush();
file.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
要从JSON文件中读取数据并创建对象,你可以使用JSONObject类的静态方法fromObject(),将JSON文件的内容转换成JSONObject,然后使用JSONObject的静态方法toBean(),将JSONObject转换回Java对象。以下是一个示例:
```java
import net.sf.json.JSONObject;
import net.sf.json.JSON;
import net.sf.json.JSONArray;
import net.sf.json.JSONException;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Paths;
public class JsonExample {
public static void main(String[] args) {
// 从JSON文件中读取数据
String jsonContent = "";
try {
byte[] encoded = Files.readAllBytes(Paths.get("person.json"));
jsonContent = new String(encoded, StandardCharsets.UTF_8);
} catch (IOException e) {
e.printStackTrace();
}
// 将JSON文件内容转换成JSONObject
JSONObject jsonObject = null;
try {
jsonObject = JSONObject.fromObject(jsonContent);
} catch (JSONException e) {
e.printStackTrace();
}
// 将JSONObject转换成Java对象
Person person = (Person) JSONObject.toBean(jsonObject, Person.class);
// 打印Java对象
System.out.println(person);
}
}
```
这里我们定义了一个Person类,它有三个属性:firstName,lastName和age。在读取JSON文件时,我们首先使用Files.readAllBytes()方法将文件内容读入到一个字节数组中,然后使用new String()方法将字节数组转换成字符串。然后,我们使用JSONObject的fromObject()方法将JSON字符串转换成JSONObject对象。最后,我们使用JSONObject的toBean()方法将JSONObject对象转换成Java对象。
相关推荐
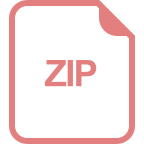
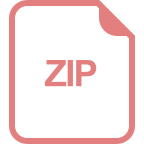










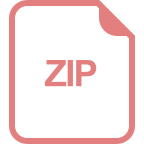
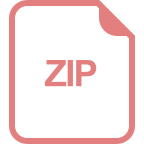
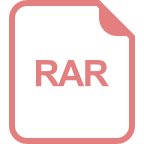
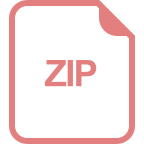
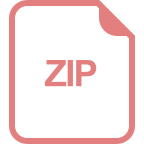