请帮我写一个python局域网软件分发的软件可以传输文件并且自动安装并且要使用Pyqt5写软件界面
时间: 2023-05-17 07:07:47 浏览: 182
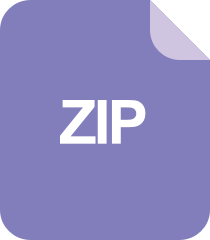
基于Python PyQt5实现的录音软件源码,带GUI界面
好的,我可以为您提供一个基于Pyqt5的局域网软件分发工具,可以传输文件并自动安装。以下是代码示例:
```python
import os
import shutil
from PyQt5.QtWidgets import QApplication, QWidget, QFileDialog, QPushButton, QLabel, QVBoxLayout, QHBoxLayout, QProgressBar
from PyQt5.QtCore import Qt, QThread, pyqtSignal
class FileTransferThread(QThread):
progress_signal = pyqtSignal(int)
def __init__(self, src_path, dest_path):
super().__init__()
self.src_path = src_path
self.dest_path = dest_path
def run(self):
# Copy file to destination path
shutil.copy(self.src_path, self.dest_path)
# Install file
os.system(self.dest_path)
# Emit progress signal
self.progress_signal.emit(100)
class FileTransferWidget(QWidget):
def __init__(self):
super().__init__()
# Create UI elements
self.file_label = QLabel("请选择要传输的文件:")
self.file_button = QPushButton("选择文件")
self.dest_label = QLabel("请选择目标路径:")
self.dest_button = QPushButton("选择路径")
self.transfer_button = QPushButton("开始传输")
self.progress_bar = QProgressBar()
self.progress_bar.setValue(0)
# Connect signals and slots
self.file_button.clicked.connect(self.select_file)
self.dest_button.clicked.connect(self.select_dest)
self.transfer_button.clicked.connect(self.start_transfer)
# Create layout
file_layout = QHBoxLayout()
file_layout.addWidget(self.file_label)
file_layout.addWidget(self.file_button)
dest_layout = QHBoxLayout()
dest_layout.addWidget(self.dest_label)
dest_layout.addWidget(self.dest_button)
button_layout = QHBoxLayout()
button_layout.addWidget(self.transfer_button)
main_layout = QVBoxLayout()
main_layout.addLayout(file_layout)
main_layout.addLayout(dest_layout)
main_layout.addLayout(button_layout)
main_layout.addWidget(self.progress_bar)
self.setLayout(main_layout)
# Initialize variables
self.src_path = ""
self.dest_path = ""
def select_file(self):
# Open file dialog to select file
file_dialog = QFileDialog()
file_dialog.setFileMode(QFileDialog.ExistingFile)
file_dialog.setNameFilter("Python files (*.py)")
if file_dialog.exec_():
self.src_path = file_dialog.selectedFiles()[0]
self.file_label.setText("已选择文件:" + self.src_path)
def select_dest(self):
# Open file dialog to select destination path
dest_dialog = QFileDialog()
dest_dialog.setFileMode(QFileDialog.Directory)
if dest_dialog.exec_():
self.dest_path = dest_dialog.selectedFiles()[0]
self.dest_label.setText("已选择目标路径:" + self.dest_path)
def start_transfer(self):
# Check if source and destination paths are selected
if not self.src_path or not self.dest_path:
return
# Create file transfer thread
self.transfer_thread = FileTransferThread(self.src_path, self.dest_path)
self.transfer_thread.progress_signal.connect(self.update_progress)
# Start file transfer thread
self.transfer_thread.start()
def update_progress(self, progress):
# Update progress bar
self.progress_bar.setValue(progress)
if __name__ == "__main__":
app = QApplication([])
widget = FileTransferWidget()
widget.show()
app.exec_()
```
您可以使用该工具选择要传输的文件和目标路径,然后点击“开始传输”按钮即可自动传输和安装文件。传输过程中,进度条会显示传输进度。
阅读全文
相关推荐
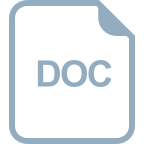
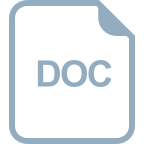
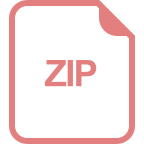
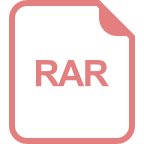
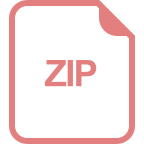
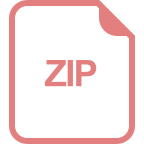
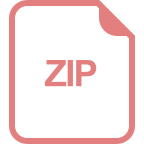
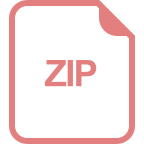
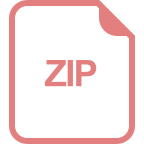
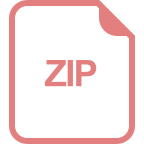
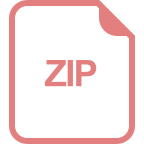
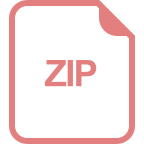
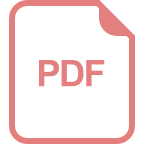
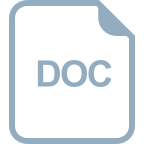
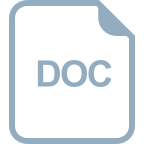
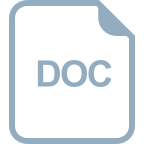