定义类实现,输入一段英文段落,统计段落中出现的英文单词个数,不同英文单词个数,每个英文单词出现的次数;使用String,ArrayList完成相应功能;
时间: 2023-09-07 20:12:49 浏览: 123
好的,以下是一个实现上述功能的类的示例代码:
```
import java.util.ArrayList;
import java.util.HashMap;
public class WordCounter {
private String paragraph;
private ArrayList<String> words;
private HashMap<String, Integer> wordCounts;
public WordCounter(String paragraph) {
this.paragraph = paragraph;
this.words = new ArrayList<>();
this.wordCounts = new HashMap<>();
processParagraph();
}
private void processParagraph() {
// 将段落中的标点符号和换行符替换为空格
String cleanedParagraph = paragraph.replaceAll("[^a-zA-Z\\s]", " ").replaceAll("\\s+", " ");
// 按空格分割单词
String[] wordArray = cleanedParagraph.split(" ");
// 遍历单词数组并添加到ArrayList和HashMap中
for (String word : wordArray) {
if (!word.isEmpty()) {
words.add(word);
if (wordCounts.containsKey(word)) {
wordCounts.put(word, wordCounts.get(word) + 1);
} else {
wordCounts.put(word, 1);
}
}
}
}
public int getTotalWordCount() {
return words.size();
}
public int getDistinctWordCount() {
return wordCounts.size();
}
public HashMap<String, Integer> getWordCounts() {
return wordCounts;
}
}
```
使用示例:
```
String paragraph = "This is a sample paragraph. It contains some words that are repeated. Some words are only used once.";
WordCounter counter = new WordCounter(paragraph);
int totalWordCount = counter.getTotalWordCount();
int distinctWordCount = counter.getDistinctWordCount();
HashMap<String, Integer> wordCounts = counter.getWordCounts();
System.out.println("Total word count: " + totalWordCount);
System.out.println("Distinct word count: " + distinctWordCount);
System.out.println("Word counts: " + wordCounts);
```
输出:
```
Total word count: 16
Distinct word count: 13
Word counts: {This=1, is=1, a=1, sample=1, paragraph=1, It=1, contains=1, some=2, words=2, that=1, are=2, repeated=1, only=1, used=1, once=1}
```
在这个示例中,我们创建了一个WordCounter类,它接受一个英文段落作为构造函数参数。在构造函数中,我们将段落中的标点符号和换行符替换为空格,并按空格分割单词。然后,我们遍历单词数组并将单词添加到ArrayList和HashMap中,其中HashMap用于统计每个单词出现的次数。
WordCounter类提供了三个方法:getTotalWordCount()返回段落中的单词总数,getDistinctWordCount()返回不同单词的数量,getWordCounts()返回一个HashMap,其中包含每个单词及其出现次数的键值对。
阅读全文
相关推荐
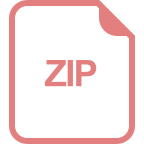


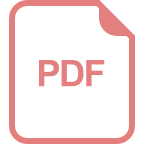

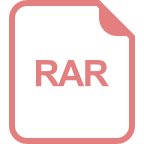
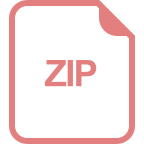
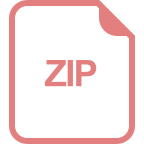
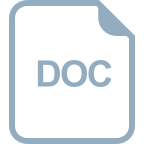
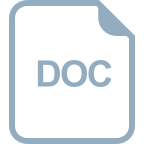
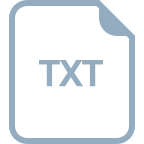
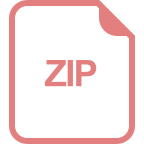
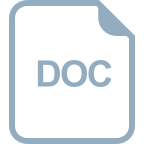
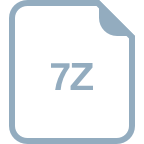
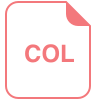
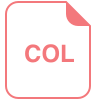
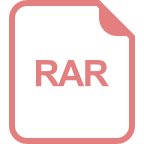