c++文件的输入输出流
时间: 2023-12-13 17:02:51 浏览: 51
在 C++ 中,我们可以使用输入输出流来读取和写入文件。常用的输入输出流有 `ifstream` 和 `ofstream`。
`ifstream` 用于从文件中读取数据,`ofstream` 用于向文件中写入数据。
### ifstream
以下是一个示例,演示如何使用 `ifstream` 从文件中读取数据:
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
ifstream inputFile("example.txt");
if (!inputFile) {
cerr << "File open failed" << endl;
return 1;
}
string line;
while (getline(inputFile, line)) {
cout << line << endl;
}
inputFile.close();
return 0;
}
```
在上面的示例中,我们首先创建了一个 `ifstream` 对象 `inputFile`,并将文件名 `example.txt` 作为参数传递给它。然后,我们使用 `getline` 函数逐行读取文件,将每一行存储在 `string` 类型的变量 `line` 中,并将其输出到控制台。
最后,我们关闭了文件。
### ofstream
以下是一个示例,演示如何使用 `ofstream` 将数据写入文件:
```cpp
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ofstream outputFile("example.txt");
if (!outputFile) {
cerr << "File open failed" << endl;
return 1;
}
outputFile << "Hello, world!" << endl;
outputFile << "This is a test." << endl;
outputFile.close();
return 0;
}
```
在上面的示例中,我们首先创建了一个 `ofstream` 对象 `outputFile`,并将文件名 `example.txt` 作为参数传递给它。然后,我们使用 `<<` 运算符将数据写入文件。
最后,我们关闭了文件。
相关推荐
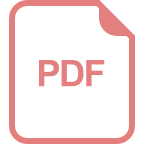
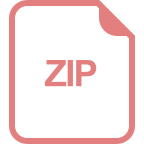
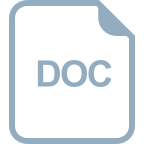
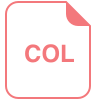
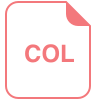
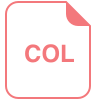
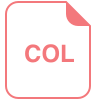
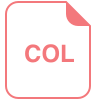









