Uniapp实现评论功能怎么写完整代码
时间: 2024-03-29 13:38:58 浏览: 77
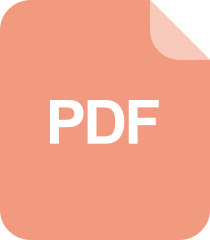
uni-app实现点赞评论功能
为了实现完整的评论功能,需要在前端和后端都编写相应的代码。
1. 在前端部分,我们需要编写评论组件的HTML、CSS和JavaScript代码,并调用后端API实现评论的提交和加载功能。
下面是一个基于uniCloud云函数实现评论功能的UniApp组件代码示例:
```html
<template>
<div>
<textarea v-model="content"></textarea>
<button @click="submitComment">提交评论</button>
<ul>
<li v-for="(comment, index) in comments" :key="comment._id">
{{ comment.content }}
</li>
</ul>
</div>
</template>
<script>
import { uniCloud } from '@/utils/request'
export default {
data() {
return {
content: '',
comments: []
}
},
methods: {
async submitComment() {
if (!this.content) {
uni.showToast({
title: '请输入评论内容',
icon: 'none'
})
return
}
try {
const res = await uniCloud.callFunction({
name: 'comment',
data: {
action: 'create',
content: this.content
}
})
if (res.result.code === 0) {
uni.showToast({
title: '评论成功'
})
this.content = ''
this.loadComments()
} else {
uni.showToast({
title: '评论失败',
icon: 'none'
})
}
} catch (err) {
uni.showToast({
title: '评论失败',
icon: 'none'
})
}
},
async loadComments() {
try {
const res = await uniCloud.callFunction({
name: 'comment',
data: {
action: 'list'
}
})
if (res.result.code === 0) {
this.comments = res.result.data
} else {
uni.showToast({
title: '加载评论失败',
icon: 'none'
})
}
} catch (err) {
uni.showToast({
title: '加载评论失败',
icon: 'none'
})
}
}
},
mounted() {
// 加载评论列表
this.loadComments()
}
}
</script>
<style>
textarea {
width: 100%;
height: 80px;
}
</style>
```
在上面的代码中,我们定义了一个 `comment` 组件,包括一个 `textarea` 输入框、一个 `button` 按钮和一个 `ul` 列表,用于展示评论列表。其中,`v-model` 指令绑定了 `content` 变量,`@click` 事件绑定了 `submitComment` 方法,`v-for` 指令渲染了评论列表。
在 `submitComment` 方法中,我们使用了uniCloud云函数的API调用方法,通过传递 `action` 和 `content` 参数调用了后端的评论创建接口,成功后清空输入框并重新加载评论列表。在 `loadComments` 方法中,我们同样使用了uniCloud云函数的API调用方法,通过传递 `action` 参数调用了后端的评论列表接口,成功后更新了 `comments` 变量。
2. 在后端部分,我们需要编写评论相关的云函数和数据库操作代码,用于处理前端的API请求。
下面是一个基于uniCloud云函数实现评论功能的后端代码示例:
```js
'use strict'
const db = uniCloud.database()
const collection = db.collection('comment')
exports.main = async (event, context) => {
const { action, content } = event
switch (action) {
case 'create':
return createComment(content)
case 'list':
return listComments()
default:
return {
code: -1,
message: '非法请求'
}
}
}
async function createComment(content) {
try {
const res = await collection.add({
content,
createdAt: new Date().getTime()
})
return {
code: 0,
message: '评论成功',
data: res
}
} catch (err) {
console.error(err)
return {
code: -1,
message: '评论失败'
}
}
}
async function listComments() {
try {
const res = await collection.orderBy('createdAt', 'desc').get()
return {
code: 0,
message: '加载评论成功',
data: res.data
}
} catch (err) {
console.error(err)
return {
code: -1,
message: '加载评论失败'
}
}
}
```
在上面的代码中,我们定义了一个 `comment` 云函数,包括了 `createComment` 和 `listComments` 两个函数。`createComment` 函数用于创建评论,将评论内容和创建时间写入到数据库中;`listComments` 函数用于获取评论列表,按照创建时间倒序排列并返回给前端。
需要注意的是,由于评论功能涉及到用户隐私和数据安全问题,我们需要在后端进行必要的数据校验和用户身份验证,避免不良信息的传播和恶意攻击的发生。
阅读全文
相关推荐
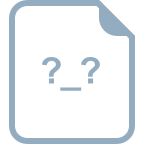
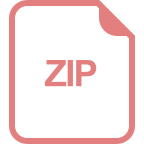
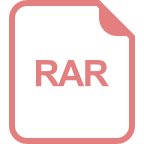
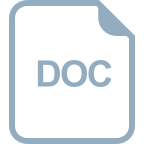
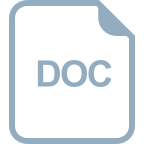
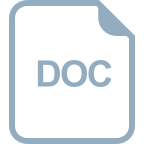
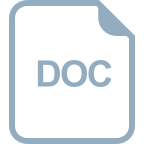
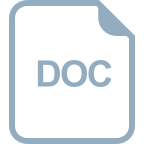








