通过实体类生成建表sql
时间: 2023-03-30 19:03:20 浏览: 719
可以使用Java Persistence API (JPA)的工具来生成建表SQL语句,具体步骤如下:
1. 在实体类上添加注解,如@Entity、@Table等,用于指定表名、字段名等信息。
2. 使用JPA的工具,如Hibernate、EclipseLink等,生成建表SQL语句。
3. 根据生成的SQL语句,在数据库中创建对应的表。
需要注意的是,生成的SQL语句可能不完全符合需求,需要根据实际情况进行调整。
相关问题
springboot实现自定义sql生成建表工具
Spring Boot并不提供自定义SQL生成建表工具,但是可以通过使用JPA(Java Persistence API)实现这个功能。JPA是一种Java规范,它定义了对象-关系映射(ORM)的标准,可以将Java对象映射到关系型数据库中的表结构。
下面是一个简单的示例,演示如何使用Spring Boot和JPA来生成建表SQL:
1. 添加依赖
在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>${hibernate.version}</version>
</dependency>
```
2. 配置数据源
在application.properties文件中配置数据源:
```
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=root
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
```
3. 实体类定义
定义一个实体类,使用JPA注解指定表名和字段名:
```
@Entity
@Table(name = "user")
public class UserEntity {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String name;
private String email;
// getters and setters
}
```
4. 生成建表SQL
使用Hibernate提供的SchemaExport工具生成建表SQL:
```
@Configuration
public class JpaConfig {
@Autowired
private EntityManagerFactory entityManagerFactory;
@Bean
public CommandLineRunner commandLineRunner() {
return args -> {
SchemaExport schemaExport = new SchemaExport();
schemaExport.setFormat(true);
schemaExport.setDelimiter(";");
schemaExport.setOutputFile("schema.sql");
schemaExport.execute(EnumSet.of(TargetType.SCRIPT), SchemaExport.Action.CREATE, entityManagerFactory);
};
}
}
```
这段代码定义了一个CommandLineRunner bean,它会在应用启动时执行。在执行过程中,它使用Hibernate的SchemaExport工具生成建表SQL,并将其输出到文件schema.sql中。
5. 运行应用程序
现在,您可以运行应用程序并查看生成的建表SQL。如果您使用的是默认配置,建表SQL将输出到项目根目录下的schema.sql文件中。
以上就是使用Spring Boot和JPA生成建表SQL的简单示例。当然,实际上,您可能需要更复杂的实体类和更复杂的数据库模式,但这个示例可以为您提供一个好的起点。
java根据实体类对象生成mysql建表语句
在Java中,根据实体类(通常使用ORM框架如Hibernate、MyBatis等)生成MySQL建表语句的过程称为数据映射或逆工程。这个过程通常是自动化的,通过分析Java实体类的字段、属性以及它们的数据类型、约束等信息来构建SQL创建表的DDL(Data Definition Language)语句。
例如,假设有一个名为`User`的Java实体类,它有`id`(int,主键)、`name`(String,非空)和`email`(String,唯一)等字段,对应的MySQL建表语句可能会像这样:
```sql
CREATE TABLE `User` (
`id` INT NOT NULL AUTO_INCREMENT,
`name` VARCHAR(255) NOT NULL,
`email` VARCHAR(255) UNIQUE NOT NULL,
PRIMARY KEY (`id`)
);
```
要自动生成这样的SQL,你可以使用特定工具,比如Hibernate的`hbm2ddl`工具,或者MyBatis Generator等插件。具体的命令行语法或配置会根据所使用的工具有所不同。
阅读全文
相关推荐
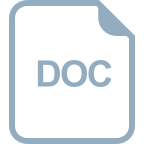
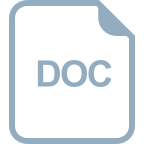
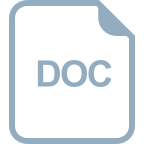
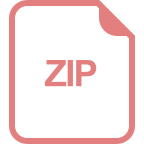
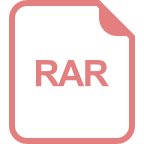



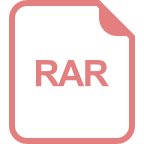
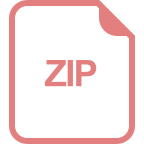
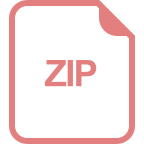
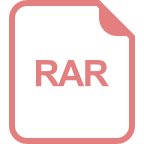
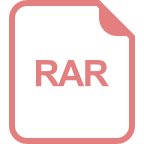
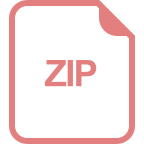
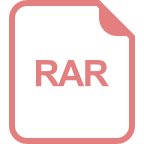
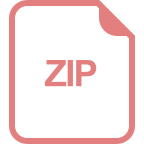
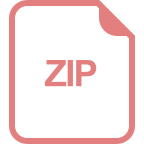