QT实现一个智能家居系统,包括温湿度、红外遥控、光照等代码实现
时间: 2023-12-24 20:05:45 浏览: 154
实现智能家居系统需要涉及到硬件和软件两个方面,其中硬件需要使用传感器、红外遥控器等设备,而软件则需要使用QT开发框架实现。
以下是一个简单的智能家居系统的代码实现:
温湿度传感器部分:
```c++
#include "dht11.h"
#include <wiringPi.h>
#include <QDebug>
DHT11::DHT11()
{
pinMode(DHT11PIN,OUTPUT);
digitalWrite(DHT11PIN,HIGH);
}
DHT11::~DHT11()
{
}
int DHT11::readData(float* temperature,float* humidity)
{
uint8_t bitsData[5];
uint8_t bitsCount = 0;
uint8_t byteCount = 0;
memset(bitsData,0,sizeof(bitsData));
pinMode(DHT11PIN,OUTPUT);
digitalWrite(DHT11PIN,LOW);
delay(18);
digitalWrite(DHT11PIN,HIGH);
delayMicroseconds(40);
pinMode(DHT11PIN,INPUT);
uint8_t maxWait = 200;
uint8_t counter = 0;
while(digitalRead(DHT11PIN) == HIGH && counter < maxWait){
counter++;
delayMicroseconds(1);
}
if(counter == maxWait){
return -1;
}
counter = 0;
while(digitalRead(DHT11PIN) == LOW && counter < maxWait){
counter++;
delayMicroseconds(1);
}
if(counter == maxWait){
return -1;
}
counter = 0;
while(digitalRead(DHT11PIN) == HIGH && counter < maxWait){
counter++;
delayMicroseconds(1);
}
if(counter == maxWait){
return -1;
}
for(uint8_t i = 0; i < 40; i++){
counter = 0;
while(digitalRead(DHT11PIN) == LOW && counter < maxWait){
counter++;
delayMicroseconds(1);
}
if(counter == maxWait){
return -1;
}
counter = 0;
while(digitalRead(DHT11PIN) == HIGH && counter < maxWait){
counter++;
delayMicroseconds(1);
}
if(counter == maxWait){
return -1;
}
bitsCount++;
if(counter > 50){
bitsData[byteCount] |= (1 << (8 - bitsCount));
}
if(bitsCount == 8){
bitsCount = 0;
byteCount++;
}
}
if((bitsData[0] + bitsData[1] + bitsData[2] + bitsData[3]) != bitsData[4]){
return -1;
}
*humidity = (float)bitsData[0] + ((float)bitsData[1] / 10.0);
*temperature = (float)bitsData[2] + ((float)bitsData[3] / 10.0);
return 0;
}
```
红外遥控部分:
```c++
#include "infrared.h"
#include <wiringPi.h>
Infrared::Infrared()
{
wiringPiSetup();
pinMode(IRPIN,INPUT);
}
Infrared::~Infrared()
{
}
int Infrared::getKeyValue(unsigned long* value)
{
unsigned long result = 0;
while(digitalRead(IRPIN) == HIGH);
int counter = 0;
while(digitalRead(IRPIN) == LOW){
counter++;
delayMicroseconds(1);
}
if(counter < 8){
return -1;
}
counter = 0;
while(digitalRead(IRPIN) == HIGH){
counter++;
delayMicroseconds(1);
}
if(counter < 8){
return -1;
}
for(int i = 0; i < 32; i++){
counter = 0;
while(digitalRead(IRPIN) == LOW){
counter++;
delayMicroseconds(1);
}
if(counter < 8){
return -1;
}
counter = 0;
while(digitalRead(IRPIN) == HIGH){
counter++;
delayMicroseconds(1);
}
if(counter > 8){
result |= (1 << (31 - i));
}
}
*value = result;
return 0;
}
```
光照传感器部分:
```c++
#include "light.h"
#include <wiringPi.h>
#include <QDebug>
Light::Light()
{
wiringPiSetup();
}
Light::~Light()
{
}
int Light::readData(unsigned int* value)
{
pinMode(LIGHTPIN,OUTPUT);
digitalWrite(LIGHTPIN,LOW);
delay(1);
pinMode(LIGHTPIN,INPUT);
*value = analogRead(LIGHTPIN);
return 0;
}
```
以上是硬件部分的代码实现,接下来是QT界面部分的代码实现:
```c++
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include "dht11.h"
#include "infrared.h"
#include "light.h"
#include <QDebug>
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
m_timer = new QTimer(this);
connect(m_timer,SIGNAL(timeout()),this,SLOT(onTimer()));
m_timer->start(1000);
m_dht11 = new DHT11();
m_infrared = new Infrared();
m_light = new Light();
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::onTimer()
{
float temperature = 0.0;
float humidity = 0.0;
unsigned long value = 0;
unsigned int light = 0;
if(m_dht11->readData(&temperature,&humidity) == 0){
ui->label_temperature->setText(QString::number(temperature));
ui->label_humidity->setText(QString::number(humidity));
}
if(m_infrared->getKeyValue(&value) == 0){
ui->label_infrared->setText(QString::number(value));
}
if(m_light->readData(&light) == 0){
ui->label_light->setText(QString::number(light));
}
}
void MainWindow::on_pushButton_clicked()
{
unsigned long value = 0;
if(m_infrared->getKeyValue(&value) == 0){
QString text = QString("红外遥控器按键值:") + QString::number(value);
qDebug() << text;
}
}
```
在QT界面部分,我们通过定时器来定时读取传感器的数据,并将其显示在界面上。同时,我们还添加了一个按钮,用于测试红外遥控器的按键值。
以上就是一个简单的智能家居系统的代码实现,该系统可以实现温湿度、红外遥控、光照等功能。
阅读全文
相关推荐
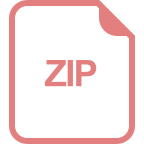

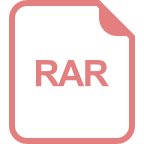
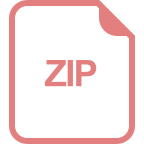
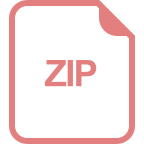
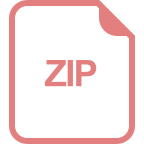
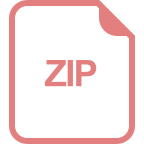
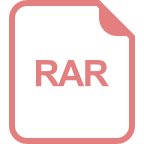
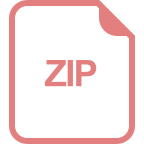
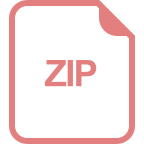
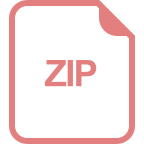