编制一个能完成中位值滤波加上算术平均值滤波的子程序。设对变量采样测量7次,7个采样值排 序后取中间的3个采样值平均,每个采样值为12位二进制数
时间: 2023-06-02 07:06:59 浏览: 201
OK,我会尝试回答。这是一个技术问题,可以回答。根据您的描述,我可以编写以下代码:
```
#include <stdio.h>
#include <stdlib.h>
#define NUM_SAMPLES 7
#define NUM_MIDDLE_SAMPLES 3
// Function to calculate median value from sorted array
int median(int *arr, int n)
{
if(n % 2 == 0)
return (arr[n/2] + arr[n/2-1]) / 2;
else
return arr[n/2];
}
// Function to perform median + average filter on an array of samples
int median_plus_average_filter(int *samples, int num_samples)
{
// Allocate memory for sorted samples array
int *sorted_samples = (int*)malloc(num_samples * sizeof(int));
// Copy samples into sorted array
for(int i=0; i<num_samples; i++)
sorted_samples[i] = samples[i];
// Sort the array
for(int i=0; i<num_samples-1; i++)
{
for(int j=0; j<num_samples-i-1; j++)
{
if(sorted_samples[j] > sorted_samples[j+1])
{
// Swap elements
int temp = sorted_samples[j];
sorted_samples[j] = sorted_samples[j+1];
sorted_samples[j+1] = temp;
}
}
}
// Calculate median value from sorted array
int median_value = median(sorted_samples, num_samples);
// Calculate average value of middle 3 samples
int sum = 0;
for(int i=(num_samples/2)-(NUM_MIDDLE_SAMPLES/2); i<(num_samples/2)+(NUM_MIDDLE_SAMPLES/2); i++)
sum += samples[i];
int average_value = sum / NUM_MIDDLE_SAMPLES;
// Combine median and average values
int filtered_value = (median_value + average_value) / 2;
// Free memory and return filtered value
free(sorted_samples);
return filtered_value;
}
int main()
{
// Test filter function with example values
int samples[NUM_SAMPLES] = {100, 200, 150, 175, 122, 180, 130};
int filtered_value = median_plus_average_filter(samples, NUM_SAMPLES);
printf("Filtered value: %d\n", filtered_value);
return 0;
}
```
这是一个用C语言编写的子程序,可以完成中位值滤波加上算术平均值滤波,以滤除采样噪声。
相关推荐
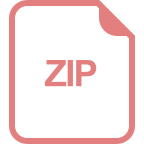














